How to get full list of Unity shader properties
Solution 1
Unity has it's own shader syntax called ShaderLab.
All the necessary information about it can be found on this website.
As for the properties, check out this link.
As nvidia is not supporting CG anymore, latest unity versions actually compile shaders using HLSL compiler and transform the resulting bytecode to GLSL. The CG shader code continues to work mostly unchanged. Nowadays you can take advantage of modern shader features, like compute shaders and tessellation, that weren't supported by CG, using HLSL syntax.
For example these shader properties:
_MyColor ("Some Color", Color) = (1,1,1,1)
_MyVector ("Some Vector", Vector) = (0,0,0,0)
_MyRange ("My Range", Range (0, 1)) = 1
_MyFloat ("My float", Float) = 0.5
_MyInt ("My Int", int) = 1
_MyTexture2D ("Texture2D", 2D) = "white" {}
_MyTexture3D ("Texture3D", 3D) = "white" {}
_MyCubemap ("Cubemap", CUBE) = "" {}
would be declared for access in Cg/HLSL code as:
fixed4 _MyColor;
float4 _MyVector;
float _MyRange;
float _MyFloat;
int _MyInt;
sampler2D _MyTexture2D;
sampler3D _MyTexture3D;
samplerCUBE _MyCubemap;
Property types in ShaderLab map to Cg/HLSL variable types this way:
• Color and Vector properties map to float4, half4 or fixed4 variables.
• Range and Float properties map to float, half or fixed variables.
• Texture properties map to sampler2D variables for regular (2D) textures.
• Cubemaps map to samplerCUBE.
• 3D textures map to sampler3D.
Solution 2
The properties of a shader in Unity are just a public variables visible to the engine, because Cg shader is wrapped into ShaderLab program.
You can see the structure of a shader in Unity at this presentation of Andy Touch: https://www.youtube.com/watch?v=zr1zQpdYG1Q&t=7m36s
So, when you see
fixed _Stroke;
half4 _StrokeColor;
later in your code it's just an actual variables that are used by Cg program and binded to these properties.
Check adjacent Unity docs section to get to know how these properties are mapped to shader variables.
Related videos on Youtube
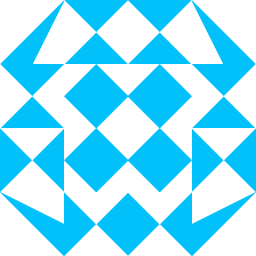
Nullititiousness
Updated on June 04, 2022Comments
-
Nullititiousness 12 months
I am quite new to shaders and have been searching for a full list of Unity shader properties. I haven't found any such documentation. What I found was SL-Properties. Where can I find a full list of properties and their functions?
UPDATE
An example was given in SL-Properties showing the list of properties for a water shader namely,
_WaveScale
,_Fresnel
,_BumpMap
and so on. Knowing these specific properties make it easier to arrive at a solution. I recently tried coding something similar to a stroke before I found out about the following properties.fixed _Stroke; half4 _StrokeColor;
-
Daniel about 5 yearshow should I declare an uint in both properties and cg? I tried exactly like _MyInt (changing the type from int to uint, obviously) but it didn't compile.
-
Admin almost 5 yearsYou haven't really answered the question: where can one find a full list of the shader properties?