How to get JSON array from file with getJSON?
Solution 1
$.getJSON
is an asynchronous function, so returning something inside that function does nothing, as it's not in scope, or received yet. You should probably do something like:
function getArray(){
return $.getJSON('questions.json');
}
getArray().done(function(json) {
// now you can use json
var questions = [];
$.each(json, function(key, val) {
questions[key] = { Category: val.Category };
});
});
Solution 2
Your conditional within the for
loop prevents anything from being added to your array. Instead, check if your json object has the property, then get the value and add it to your array. In other words:
if (questions.hasOwnProperty(key))
should be if (json.hasOwnProperty(key))
Also, you can't simply return
the result of an AJAX call like that, because the method runs asynchronously. That return
is actually applied to the inner success
function callback, not getArray
. You have to use a callback pattern in order to pass the data only once it has been received, and operate on it accordingly.
(Of course since the array is defined in the outer scope you wouldn't have to return it anyway, but if you attempted to use it before the AJAX method ended it would be empty.)
Assuming you are going to render it to the DOM using a method called renderJSON
:
var questions = [];
function getArray(){
$.getJSON('questions.json', function (json) {
for (var key in json) {
if (json.hasOwnProperty(key)) {
var item = json[key];
questions.push({
Category: item.Category
});
}
}
renderJSON(questions);
});
}
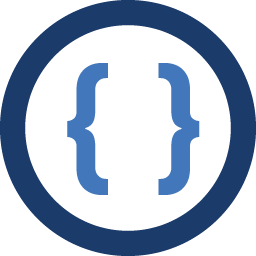
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
How could I get an array json of a json file with javascript and jquery
I was triyng with the next code, with it doesnt work:
var questions = []; function getArray(){ $.getJSON('questions.json', function (json) { for (var key in json) { if (json.hasOwnProperty(key)) { var item = json[key]; questions.push({ Category: item.Category }); } } return questions; }) }
this is the json file called: questions.json
{ "Biology":{ "Category":{ "cell":{ "question1":{ "que1":"What is the cell?" }, "option1":{ "op1":"The cell is the basic structural and functional unit", "op2":"is a fictional supervillain in Dragon Ball" }, "answer1":"opt1" } } }, "Astronomy":{ "Category":{ "Mars":{ "question1":{ "que1":"How many moons does Mars?" }, "option1":{ "op1":"5", "op2":"2" }, "answer1":"opt2" } } } }
I want to get an array with this format {Biology:{Category:{cell:{question1....}}}}
-
nbrooks about 11 years@chikatetsu Works for me: jsfiddle.net/H2jQD. What do you mean by 'not working'? What do you expect it to do?
-
nbrooks about 11 yearsP.S. @whoeverdownvoted It's always good to explain why...OP changed code after my post, hence your not being able to see the old problem.
-
Admin about 11 yearsBy the way how could i get the value for example of Astronomy?? console.log(questions[1].[Astronomy]), on this way is ok?
-
Admin about 11 yearstry it console.log(questions[1][Category])
-
adeneo about 11 yearsDid you try
questions.Astronomy
? Using the key that way actually makes into an object, so push() would be the way to go if you wanted a number indexed array.