How to get parameter value from react-router-dom v6 in class
Solution 1
The library-provided HOC, withRouter, has been deprecated in React Router v6. If you need to use v6 and are using class-based React components, then you will need to write your own HOC which wraps the v6 use* hooks.
For example:
export function withRouter( Child ) {
return ( props ) => {
const location = useLocation();
const navigate = useNavigate();
return <Child { ...props } navigate={ navigate } location={ location } />;
}
}
This is useful if you have a large code base that you need to move to v6, but haven't migrated all of your class-based components into functional ones.
This is based on the recommendation from the dev team as stated in this Github issue: https://github.com/ReactTraining/react-router/issues/7256 t
Solution 2
Typescript HoC
import React from "react";
import { NavigateFunction, useLocation, useNavigate, useParams } from "react-router";
export interface RoutedProps<Params = any, State = any> {
location: State;
navigate: NavigateFunction;
params: Params;
}
export function withRouter<P extends RoutedProps>( Child: React.ComponentClass<P> ) {
return ( props: Omit<P, keyof RoutedProps> ) => {
const location = useLocation();
const navigate = useNavigate();
const params = useParams();
return <Child { ...props as P } navigate={ navigate } location={ location } params={ params }/>;
}
}
then let the class component props extends RoutedProps with optional Params and State types
Related videos on Youtube
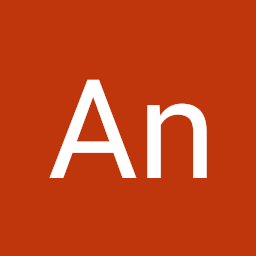
An Dương
Updated on June 04, 2022Comments
-
An Dương almost 2 years
I'm using react-router-dom v6 and don't know how to get parameter value
ex:
http://localhost:3000/user/:id
i want to get
:id
Someone use hook
useParams
to get but i'm using class so i can't use hook. -
An Dương almost 4 yearsi'm using react-router-dom v6 and withRouter is not available:
'withRouter' is not exported from 'react-router-dom'
-
Red Baron almost 4 years@AnDương is there a reason you need to use v6?
-
An Dương almost 4 yearsyes, it is very light and have Outlet is very useful
-
Red Baron almost 4 yearsand the reason you can't use a functional component?
-
romor over 2 yearsShouldn't
location
be of typeLocation
(as exported fromreact-router
) andparams
of typeURLSearchParams
?