How to get parameters from the Angular ActivatedRoute
11,139
Solution 1
Change to this.id = +params.get('id')
.
You should use the method get()
because it returns a single value for the given parameter id
.
You were getting an error because params
is not a key with id
as a value.
export class RecipeEditComponent implements OnInit {
id: number;
editMode = false;
constructor(private route: ActivatedRoute) { }
ngOnInit() {
// paramMap replaces params in Angular v4+
this.route.paramMap.subscribe(params: ParamMap => {
this.id = +params.get('id');
console.log(this.id);
});
}
Solution 2
The problem was in the route definition: putting : instead of ::
Solution 3
I think the following code is going to work in your case:
ngOnInit() {
this.heroes$ = this.route.paramMap.pipe(
switchMap(params => {
// (+) before `params.get()` turns the string into a number
this.selectedId = +params.get('id');
return this.service.getHeroes();
})
);
}
Also you can try this:
this.sessionId = this.route
.queryParamMap
.pipe(map(params => params.get('id') || 'None'));
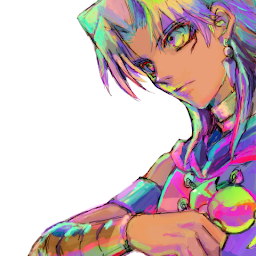
Author by
faouzi Ch
Updated on June 05, 2022Comments
-
faouzi Ch almost 2 years
I'm trying to get the prameter :id from my activated route using observables. When I print params on the console I get the right values for :id. But it's not the case for this.id. I get the value NaN. Can you tell me what is the problem
export class RecipeEditComponent implements OnInit { id: number; editMode = false; constructor(private route: ActivatedRoute) { } ngOnInit() { this.route.params.subscribe( (params: {id: string}) => { this.id = +params.id; console.log(this.id); } ); } }
-
penleychan about 5 yearsCould you put that code into your question as code block and not as image.
-
robert about 5 yearswhat you get if you console.log(params.id)?
-
faouzi Ch about 5 yearsUndifined Even though I'm getting the right object when I print params the object with :id = 1
-
robert about 5 yearshow do you call this route? what is in your browsers address bar?
-
faouzi Ch about 5 yearsthe route is : { path: '::id/edit', component: RecipeEditComponent} The link in the browser is: localhost:4200/recipes/0/edit
-
robert about 5 yearsIs double colon :: a typo? Try with single one.
-
faouzi Ch about 5 years@robert1 yes this was the problem thanks
-
-
faouzi Ch about 5 yearsit shows this error: ERROR TypeError: "params.get is not a function"
-
Damien about 5 yearsIf you are using Angular v4+, change
params
toparamMap
. -
faouzi Ch about 5 yearsI've changed it to paramMap but now it does print nothing on the console
-
Damien about 5 yearsDo you have the
console.log(this.id)
in the code? -
faouzi Ch about 5 yearsYes, now it prints 0 as value for 'this.id' But when I print params it gives me an object with the right values of 'id'
-
RadekF about 5 yearsmaybe try this:
Number(params["id"])
-
Ellone almost 4 yearsWhy is there a + sign ? I haven't found anything on the documentation about it.
-
Damien almost 4 years@Ellone The + sign changes the id from a string to a number.