how to get percentage of two numbers and calculate from a percentage
Solution 1
dotnet have some method for do this?
Since it's just arithmetic, every programming language has this. Generally, the calculation would be...
If you want to find "What percent of Y
is X
?", such as "What percent of 100 is 50?", then you divide X
by Y
:
var percent = x / y;
or:
50 / 100 = 0.5
Note, however, that in programming languages you do have to be concerned with data types. In C# for example, if X
and Y
are int
s then the result will be 0
, not 0.5
. This is because an int
divided by an int
is also an int
.
In that case you'd need to cast them to something like a double
to get sub-integer precision:
var percent = (double)x / (double)y;
how can calculate number of users that like that post?
That would just be the reverse. Consider the values that you do know:
- I have 100 users.
- 0.05 of the total like the post.
Then the number of users who like the post is the percentage times the total:
var numberOfLikes = percentOfLikes * totalUsers;
or:
0.05 * 100 = 5
Solution 2
This is a practical explanation with words, since you're probably not into theory that much
Looking for a percentage
Write 400 out of 10'000 as a fraction, because that is what you look for: 400/10'000 = 0.04 in one (since 10'000 is all you got, hence one). That would mean 0.04*100 in 100, or 4%.
Equivalent statements:
400/10000
0.04
4%
Any given number x in a population of y is (x/y)*100%.
Given a percentage
Note how the percent sign has two zeros in it, it literally means "per hundred". So if you do *100%, it's actually the same as multiplying by one, just another notation.
The other way around, again, think in words. Let's say 50 is 20% of someting. That means there is another 80% to the total; 50*(100/20) = 250.
Equivalent statements:
50 = 20%*x
50 = 0.2*x
250 = x
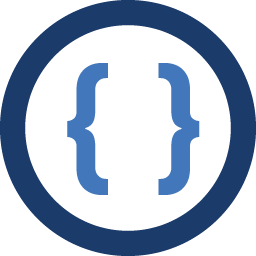
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
I am developing a application that need to calculate percentage from two numbers. dotnet have some method for do this? i like to write this calculate by myself. but i don't good in mathematics unfortunately. really, how to get percentage in mathematics? for example i have 10000 request in my application, and 400 request of them are for special part of my application. how to calculate what percent of request are request for a part of my application? and how to calculate reverse, for example, i know 5 percent of users like my special post, how can calculate number of users that like that post? Thank you and sorry for my bad English.