How to get phone number or sim card information use android studio?
Solution 1
There is no reliable way to get the phone number from the SIM card. The TelephonyManager reads the phone number from SIM card but its upto the Telecom operators to add this information in the SIM card.
Most of the Telecom operators don't add this information in SIM card hence its not reliable enough.
There is a way to use Google-Play-Service to get the phone number, but it also doesn't gurantee 100% to return the phone number. You can do it as follows.
Add following dependencies in build.gradle
:
dependencies {
...
compile 'com.google.android.gms:play-services:11.6.0'
compile 'com.google.android.gms:play-services-auth:11.6.0'
}
Create two constants in MainActivity.java
:
private static final int PHONE_NUMBER_HINT = 100;
private final int PERMISSION_REQ_CODE = 200;
In onclick
of your Button add following:
final HintRequest hintRequest =
new HintRequest.Builder().setPhoneNumberIdentifierSupported(true).build();
try {
final GoogleApiClient googleApiClient =
new GoogleApiClient.Builder(MainActivity.this).addApi(Auth.CREDENTIALS_API).build();
final PendingIntent pendingIntent =
Auth.CredentialsApi.getHintPickerIntent(googleApiClient, hintRequest);
startIntentSenderForResult(
pendingIntent.getIntentSender(),
PHONE_NUMBER_HINT,
null,
0,
0,
0
);
} catch (Exception e) {
e.printStackTrace();
}
Add onActivityResult
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == PHONE_NUMBER_HINT && resultCode == RESULT_OK) {
Credential credential = data.getParcelableExtra(Credential.EXTRA_KEY);
final String phoneNumber = credential.getId();
}
}
Solution 2
This are few methods you can use:
mTelephonyMgr = (TelephonyManager)mAppContext.getSystemService(Context.TELEPHONY_SERVICE);
String countryiso=mTelephonyMgr.getSimCountryIso();
String simOperator=mTelephonyMgr.getSimOperator();
String simOperatorName=mTelephonyMgr.getSimOperatorName();
String simSerialNo=mTelephonyMgr.getSimSerialNumber();
int simState=mTelephonyMgr.getSimState();
String subscriberID=mTelephonyMgr.getSubscriberId();
Edit
String mPhoneNumber = mTelephonyMgr.getLine1Number();
for more,visit here.
This Permission is also necessary.
<uses-permission android:name="android.permission.READ_PHONE_STATE"/>
I hope this helps.
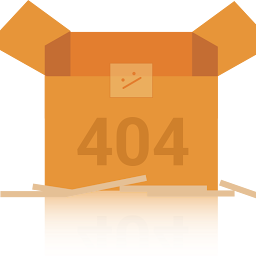
dyan tb
Updated on June 05, 2022Comments
-
dyan tb almost 2 years
How to get phone number or sim card information use android studio? (simcard 1 or 2)
I have used this code:
TelephonyManager tMgr = (TelephonyManager) getSystemService(Context.TELEPHONY_SERVICE); phone_number.setText(tMgr.getLine1Number());
And I've also added permissions in AndroidManifest:
<uses-permission android:name="android.permission.READ_PHONE_STATE"/>
But all the gadgets that I apply fail to get phone number or always generate a null value.
And this is the build.gradle I use:
dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) //noinspection GradleCompatible implementation 'com.android.support:appcompat-v7:27.1.1' implementation 'com.android.support.constraint:constraint-layout:1.1.0' //noinspection GradleCompatible implementation 'com.google.android.gms:play-services-maps:15.0.1' implementation 'com.google.android.gms:play-services-location:15.0.1' testImplementation 'junit:junit:4.12' androidTestImplementation 'com.android.support.test:runner:1.0.2' androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2' //noinspection UseOfBundledGooglePlayServices implementation 'com.google.android.gms:play-services:12.0.1' implementation 'com.google.android.gms:play-services-auth:15.0.1' }
-
dyan tb about 6 yearsSorry, this code is the same as the one I use, but the phone number I want remains null
-
dyan tb about 6 yearssorry, your code is not running on me, I also do not understand, please help me
-
dyan tb about 6 yearscannot compile, when runing in emulator or real phone
-
Sagar about 6 years@dyantb Have you updated your app level build.gradle?
-
dyan tb about 6 yearsthis code is the same as the one I use and the phone number I want remains null
-
Sagar about 6 years@dyantb Just clean and rebuild. Ensure you have internet connection to download the libraries. You are getting the compilation error because libraries are not downloaded correctly
-
Mrunal Chauhan about 6 yearsplease check from beginning,i made a few changes in beginning,and i checked it on my device,it returns proper output,and as far as i know,that number,is provided by your sim operator.if the operator is not returning that info,you will always get null. each operator has different mono poly. @dyantb
-
dyan tb about 6 yearsI've adjusted all the code with you, and keep it null, for this code (mAppContext) I have an error? so the problem is in the provider? how applications like facebook, alibaba, and other online stores deal with it?
-
Mrunal Chauhan about 6 years@dyantb you can replace mAppContext with
getApplicationContext()
. -
dyan tb about 6 yearsYour code is successful, but why every one appears only 1 phone number? My phone uses 2 sim cards, and i have 4 different sim cards and exchangeable, but that always appear only one of the sim card, although the sim card is not installed. (example: 1.provider A = 3G, 2.provider B = 4G, 3.provider C = 4G, 4.provider D = 4G and always appear is provider a)
-
Sagar about 6 years@dyantb We don't have control over the numbers shown to the user. Its some hidden mechanism used by google to predict it. Depending on their profile created by google this number is shown. As I said there is no guaranteed way to achieve this, all you can do is best case scenario
-
dyan tb almost 6 yearsthe code you mean above works on several different mobile phones, but not on my phone. how to display her phone number without a popup like that?
-
Sagar almost 6 yearsYes. They best way to get it without popup is
msisdn = mTelephonyMgr.getLine1Number();
but it also has limitation as I have mentioned in the answer. There is no way you can gurantee that you will ge the MSISDN with 100% success rate. -
dyan tb almost 6 yearsI always get a null value when using this code. how to get phone number in simcard? please help me
-
dyan tb almost 6 yearsI am still confused why applications such as social media or online shop can get the phone number usernya
-
dyan tb almost 6 yearsThanks sagar, i'm still confused as to why apps like social media or online stores can get their user's phone number. And this will bother me continuously.
-
Sagar almost 6 years@dyantb Are they working with your Phone + SIM configuration? Even Whatsapp cannot detect the phone number (if its being used for first time) on all the devices. Its always best case approach
-
dyan tb almost 6 yearsYes, I want to send the activation code via sms gateway without register and saves the user activity of the app including the phone number. you can know it from where (about Whatsapp cannot detect the phone number)?
-
Sagar almost 6 years@dyantb About Whatsapp: I tried on few test phone + SIM card (where the telecom operator didn't configure the phone number) it asked me to enter the phone number manually.
-
bugfreerammohan over 5 yearsit is working for me but how to get second sim slot number also I appreciate your answer ...help me
-
Sagar over 5 years@bugfreerammohan You can check out createForSubscriptionId . Pass in the subscription Id and you will get the TelephonyManager for that ID. once you have it, you can use
getLine1Number()
to get respective number -
bugfreerammohan over 5 yearsin some of the devices, I am getting both numbers to thank you so much
-
Radesh over 4 yearsis there any way to give numbers without dialog?
-
Krunal Patel almost 4 yearsThanks It's Work fine for me.