How to get response header in react native android?
14,624
Solution 1
you can do like this
fetchData() {
var URL_REGISTER = 'https://www.example.com';
fetch(URL_REGISTER, {method: 'POST',body: formData})
.then(
function(response) {
console.log(response.headers.get('Content-Type'));
console.log(response.headers.get('Date'));
console.log(response.status);
console.log(response.statusText);
console.log(response.type);
console.log(response.url);
if (response.status !== 200) {
console.log('Status Code: ' + response.status);
return;
}
// Examine the text in the response
response.json().then(function(data) {
console.log(data);
});
}
)
.catch(function(err) {
console.log('Fetch Error', err);
});
}
Read More about fetch: Introduction to fetch()
Solution 2
You can take the headers from response.headers
fetch(URL_REGISTER, {
method: 'POST',
body: formData
})
.then((response) => {
console.log(response.headers); //Returns Headers{} object
}
Solution 3
You should return response.json() like
return response.json();
Related videos on Youtube
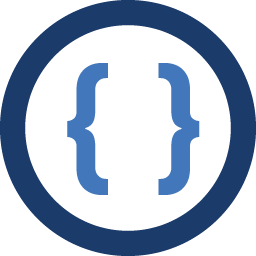
Author by
Admin
Updated on September 15, 2022Comments
-
Admin over 1 year
Hello i want to get response header after fetch POST request. I tried to debug to see what inside
response
withconsole.log(response)
. I can get response bodies fromresponseData
but i have no idea how to get the header. I want to get both header and the body. Please help. thanks:)Here's the example what i've done:
fetch(URL_REGISTER, { method: 'POST', body: formData }) .then((response) => response.json()) .then((responseData) => { if(responseData.success == 1){ this.setState({ message1: responseData.msg, }); } else{ this.setState({ message1: responseData.msg, }); } }) .done(); },
-
Admin almost 8 yearsi tried to edit like this
.then((response) => {console.log(response.headers); response.json()})
, but when i run it, it show me the error because it not readresponse.json()
. Any ideas? -
Admin almost 8 yearsThanks, that's help me a lot :)
-
Dévan Coetzee over 6 years@sptra,
.json()
returns a promise, so to get the response as JSON you need to handle the promise:.then(function (response) { console.log(response.headers); return response.json(); }).then(function (json) { console.log(json); }).catch...
-
Dévan Coetzee over 6 yearsIt is also important to note that due to W3 Standards only
Cache-Control
,Content-Language
,Content-Type
,Expires
,Last-Modified
andPragma
are accessible when making requests outside the domain.