How to get result information when updating mysql table with Doctrine ORM in symfony 1.4
Solution 1
Everything is in the doc for update and delete.
When executing DQL UPDATE and DELETE queries the executing of a query returns the number of affected rows.
See examples:
$q = Doctrine_Query::create()
->update('Account')
->set('amount', 'amount + 200')
->where('id > 200');
$rows = $q->execute();
echo $rows;
$q = Doctrine_Query::create()
->delete('Account a')
->where('a.id > 3');
$rows = $q->execute();
echo $rows;
This is related to DQL (when you are using doctrine queries). But I think ->save()
will return the current object or true/false as @PLB commented.
Solution 2
$statement = $this->entityManager->getConnection()->prepare($sql);
$statement->execute();
// the counter of rows which are updated
$counter = $statement->rowCount();
It works for me very well with doctrine 2 in symfony 2 project
Solution 3
$nrRows = $this->getEntityManager()->getConnection()->executeUpdate('UPDATE ...');
The documentation for executeUpdate:
/**
* Executes an SQL INSERT/UPDATE/DELETE query with the given parameters
* and returns the number of affected rows.
*
* This method supports PDO binding types as well as DBAL mapping types.
*
* @param string $query The SQL query.
* @param mixed[] $params The query parameters.
* @param int[]|string[] $types The parameter types.
*
* @return int The number of affected rows.
*
* @throws DBALException
*/
public function executeUpdate($query, array $params = [], array $types = [])
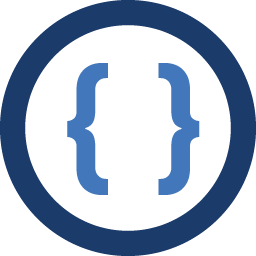
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am developing with symfony 1.4 and using Doctrine ORM. After building schema and models i've got some classes for work with database. I can also use Doctrine_query .... The only thing, that i cann`t understend is:
I need to update table.
Doctrine_Query::create()->update('table')->.....->execute().
or
$tbl = new Table(); $tbl->assignIdentifier($id); if($tbl->load()){ $tbl->setFieldname('value'); $tbl->save(); }
how can i understend was it successful result of query or not ? and how much rows was updated.
p.s. the same question is for delete operation.