How to get string value from a Java field via reflection?
Solution 1
It looks like you need a reference to an instance of the class. You would want to call get and pass in the reference, casting the return to a String.
You can use get as follows:
String strValue = (String) field.get (objectReference);
Solution 2
In ideal situations,Class
does not hold data. It merely holds the information about the structure and behavior of its instances and Instances of the Classes
hold your data to use. So your extractStringFromField
method can not extract values unless you pass any instances (from where it will actually extract values).
If the name of the parameter of the reference, you are passing to extract value is instance
, then you can easily get what you want like bellow:
String strValue = (String)field.get(instance);
Solution 3
Just usefull example code for reflection fields:
Field[] fields = InsanceName.getDeclaredFields();
for (Field field : fields) { //array for fields names
System.out.println("Fields: " + Modifier.toString(field.getModifiers())); // modyfiers
System.out.println("Fields: " + field.getType().getName()); //type var name
System.out.println("Fields: " + field.getName()); //real var name
field.setAccessible(true); //var readable
System.out.println("Fields: " + field.get(InsanceName)); //get var values
System.out.println("Fields: " + field.toString()); //get "String" values
System.out.println(""); //some space for readable code
}
Related videos on Youtube
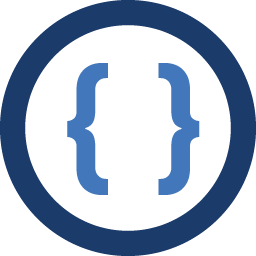
Admin
Updated on July 29, 2022Comments
-
Admin almost 2 years
I have a method:
public void extractStringFromField(Class<?> classToInspect) { Field[] allFields = classToInspect.getDeclaredFields(); for(Field field : allFields) { if(field.getType().isAssignableFrom(String.class)) { System.out.println("Field name: " + field.getName()); // How to get the actual value of the string?!?! // String strValue = ??? } } }
When this runs I get output like:
Field name: java.lang.String
Now how do I extract the actual string value into
strValue
, using reflection?-
Sazzadur Rahaman almost 11 yearsShouldn't it be?
System.out.println("Field name: " + field.getName());
-
Admin almost 11 yearsYes - cut n' paste error!
-
Sotirios Delimanolis almost 11 yearsYou want to get the value of which field? On which object?
-
Admin almost 11 yearsIf
classToInspect
is aWidget
, and theWidget
class has a String field calledfizz
, and the value of thatWidget#fizz
instance is "buzz", then I want to get thebuzz
string into an actualString
instance. -
Sotirios Delimanolis almost 11 years@TicketMonster A field only makes sense either as a static field of a Class or as an instance field. You therefore have to specify which instance it is (or
null
when it's static) with theField#get(Object)
method. Internally, it looks likeObject.Field
to retrieve the value.
-
-
Owen almost 11 yearsIt's an unfortunate aspect of the API that you need to pass in a reference even if the field is static.
-
cking24343 about 8 yearsIt also may be worth noting that you might need to make sure that the field is accessible like so: field.setAccessible(true);
-
th3morg almost 8 yearsI tried passing null and got a NullPointerException in Java 1.7.0_79
-
alvarosmh almost 4 yearsanswering to @th3morg : it works if you pass an instance ( new Foo() ) instead of null