How to get the Context from anywhere?
Solution 1
The easiest (and correct) way is:
Define a new class
public class MyApp extends Application {
private static MyApp instance;
public static MyApp getInstance() {
return instance;
}
public static Context getContext(){
return instance;
// or return instance.getApplicationContext();
}
@Override
public void onCreate() {
instance = this;
super.onCreate();
}
}
Then in your manifest you need to add this class to the "Name" field at the "Application" tab. Or edit the xml and put
<application
android:name="com.example.app.MyApp"
android:icon="@drawable/icon"
android:label="@string/app_name"
.......
<activity
......
and then from anywhere you can call
MyApp.getContext();
Hope it helps
Solution 2
Basically you have 2 types of Context - the Activity Context and the Application Context.
It's not necessary to use only one Context for everything. There's problems if you use one Context in every case you need Context. It's best to follow something like this - use Activity Context inside the Activity and the Application Context when passing a context beyond the scope of an Activity, which will help you to avoid memory leaks.
If you read this article you can see the difference between to the two Context.
The Application context will live as long as your application is alive and does not depend on the activities life cycle. If you plan on keeping long-lived objects that need a context, remember the application object.
Instead the Activity Context is associated with the activity and could be destroyed as many times as the activity is destroyed - changing screen orientation, back button etc.
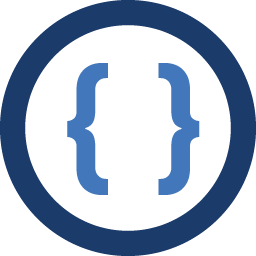
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
In Android, is there any way to get the context of the application of a static way? For example to retrieving it from a background thread.
Thanks
-
Macrosoft-Dev over 10 yearsThis will work but Just be careful as with using any singleton that you don't abuse it.Read the answer to this question explaining why the ApplicationContext is rarely (though sometimes) the right Context to use. Best Way: Unless you simply expose a public method that takes a Context as an argument inside of your classes that require Context (and pass it in from your Activity, etc), this is the way to do it. Exp: public void abc(Context c,int a); and call it from any activity.
-
ctn almost 9 yearsThis is the good answer.