How to get the Current Date in ReactNative?
Solution 1
The JavaScript runtime in React Native has access to date information just like any other environment. As such, simply:
new Date()
... will give you the current date. Here's the MDN on working with dates in JS.
If you want a good JS library to make working with dates even easier, check out MomentJS.
Solution 2
I used {new Date()}
was generating Invariant Violation: Objects are not valid
error the following date function worked for me.
const getCurrentDate=()=>{
var date = new Date().getDate();
var month = new Date().getMonth() + 1;
var year = new Date().getFullYear();
//Alert.alert(date + '-' + month + '-' + year);
// You can turn it in to your desired format
return date + '-' + month + '-' + year;//format: dd-mm-yyyy;
}
For more info https://reactnativecode.com/get-current-date-react-native-android-ios-example It works for ios as well.
Solution 3
I think this should work;
new Date().toLocaleString()
It will return date and time formatted in your local format (usually in the below format);
"6/22/2021, 2:59:00 PM"
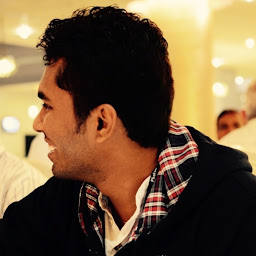
Gautham Pai
Updated on July 26, 2021Comments
-
Gautham Pai almost 3 years
I am building my first ReactNative iOS and Android app. I am an iOS coder with Swift and Obj-C. How do I fetch the current date using ReactNative.
Shall I use Native Modules or is there an ReactNative API from which I can get this data ?
-
ratsimihah over 5 yearsKeep in mind the Android JSC doesn't work well with dates, and you might need a custom JSC to handle stuffs like
toLocaleDateString
if you need internationalisation github.com/react-community/… -
RobG over 3 yearsThat will return d-m-y, there is no padding.
-
Shamiq over 3 years@RobG you can change it to your desired format and can design it as well.
-
RobG over 3 yearsTo clarify, the return statement returns d-m-y, your comment says it returns dd-mm-yyyy. It's your stated format.