How to get the current locale in Flutter using the GetX package?
Solution 1
if BuildContext is available, we can get current locale this way
Locale myLocale = Localizations.localeOf(context);
according to the documentation https://flutter.dev/docs/development/accessibility-and-localization/internationalization
another option is to use the official intl library
import 'package:intl/intl.dart';
String locale = Intl.getCurrentLocale(); // returns language tag, such as en_US
Solution 2
To get Device Current Locale:
import 'package:get/get.dart';
return GetMaterialApp(
locale: Get.deviceLocale, //returns Locale('<language code>', '<country code>')
);
To get the App Current Locale:
import 'package:get/get.dart';
return GetMaterialApp(
locale: Get.locale, //returns Locale('<language code>', '<country code>')
);
Solution 3
The authors of the package added information about this in Readme (https://pub.dev/packages/get#internationalization):
To read the system locale, you could use window.locale.
import 'dart:ui' as ui;
return GetMaterialApp(
locale: ui.window.locale,
);
Solution 4
You can use Get.locale
read only property defined inside the LocalesIntl
extension from package:get/get_utils/src/extensions/internacionalization.dart
final locale = Get.locale;
If you want current system locale, window.locale
and add import 'dart:ui';
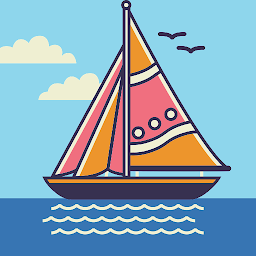
Sergey S.
Updated on December 23, 2022Comments
-
Sergey S. over 1 year
The example https://pub.dev/packages/get/example only shows setting the locale from a string manually:
void main() { runApp(GetMaterialApp( ... locale: Locale('pt', 'BR'), ...
If I don't use "locale: Locale ('pt', 'BR')", then code like Text("title".tr) don't work. So, I need setup current locale to "locale" property, but how get current locale?