How to get the displayed data of KendoGrid in json format?
Solution 1
I think you're looking for
var displayedData = $("#YourGrid").data().kendoGrid.dataSource.view()
Then stringify it as follows:
var displayedDataAsJSON = JSON.stringify(displayedData);
Hope this helps!
Solution 2
If you want to get all pages of the filtered data you can use this:
var dataSource = $("#grid").data("kendoGrid").dataSource;
var filters = dataSource.filter();
var allData = dataSource.data();
var query = new kendo.data.Query(allData);
var data = query.filter(filters).data;
Make sure to check if filters exist before trying to apply them or Kendo will complain.
Solution 3
To get count of all rows in grid
$('#YourGridName').data("kendoGrid").dataSource.total()
To get specific row items
$('#YourGridName').data("kendoGrid").dataSource.data()[1]
To get all rows in grid
$('#YourGridName').data("kendoGrid").dataSource.data()
Json to all rows in grid
JSON.stringify($('#YourGridName').data("kendoGrid").dataSource.data())
Solution 4
Something like this, to display only data that is being viewed at the moment. Also extended the grid to provide these functions all over the app.
/**
* Extends kendo grid to return current displayed data
* on a 2-dimensional array
*/
var KendoGrid = window.kendo.ui.Grid;
KendoGrid.fn.getDisplayedData = function(){
var items = this.items();
var displayedData = new Array();
$.each(items,function(key, value) {
var dataItem = new Array();
$(value).find('td').each (function() {
var td = $(this);
if(!td.is(':visible')){
//element isn't visible, don't show
return;//continues to next element, that is next td
}
if(td.children().length == 0){
//if no children get text
dataItem.push(td.text());
} else{
//if children, find leaf child, where its text is the td content
var leafElement = innerMost($(this));
dataItem.push(leafElement.text());
}
});
displayedData.push(dataItem);
});
return displayedData;
};
KendoGrid.fn.getDisplayedColumns = function(){
var grid = this.element;
var displayedColumns = new Array();
$(grid).find('th').each(function(){
var th = $(this);
if(!th.is(':visible')){
//element isn't visible, don't show
return;//continues to next element, that is next th
}
//column is either k-link or plain text like <th>Column</th>
//so we extract text using this if:
var kLink = th.find(".k-link")[0];
if(kLink){
displayedColumns.push(kLink.text);
} else{
displayedColumns.push(th.text());
}
});
return displayedColumns;
};
/**
* Finds the leaf node of an HTML structure
*/
function innerMost( root ) {
var $children = $( root ).children();
while ( true ) {
var $temp = $children.children();
if($temp.length > 0) $children = $temp;
else return $children;
}
}
Solution 5
For the JSON part, there's a helper function to extract the data in JSON format that can help:
var displayedData = $("#YourGrid").data().kendoGrid.dataSource.view().toJSON()
EDIT: after some errors with the above method due to kendo grid behavior, I found this article that solves the problem: Kendo DataSource view not always return observablearray
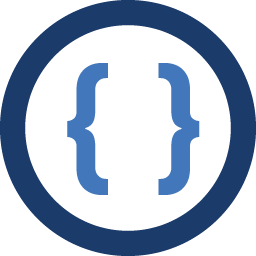
Admin
Updated on August 12, 2020Comments
-
Admin over 3 years
I have a
kendoGrid
and i would like to get theJSON
out of it after filtering and sorting how do I achieve this?something like the following,
var grid = $("#grid").data("kendoGrid"); alert(grid.dataSource.data.json); // I could dig through grid.dataSource.data and I see a function ( .json doen't exist I put it there so you know what i want to achieve )
Thanks any help is greatly appreciated!