How to get the download progress in bytes using Alamofire 4?
Solution 1
In Alamofire 4 the Progress APIs changed. All changes are explained in the Alamofire 4.0 Migration Guide.
To sum up the most important changes, that affect your use case:
// Alamofire 3
Alamofire.request(.GET, urlString, parameters: parameters, encoding: .JSON)
.progress { bytesRead, totalBytesRead, totalBytesExpectedToRead in
print("Bytes: \(bytesRead), Total Bytes: \(totalBytesRead), Total Bytes Expected: \(totalBytesExpectedToRead)")
}
can be achieved with
// Alamofire 4
Alamofire.request(urlString, method: .get, parameters: parameters, encoding: JSONEncoding.default)
.downloadProgress { progress in
print("Progress: \(progress.fractionCompleted)")
}
The returned progress
object is of the Progress
type of Apple's Foundation framework, so you can access the fractionCompleted
property.
Detailed explanation of the changes can be found in the Request Subclasses section in the Alamofire 4.0 Migration guide. The pull request 1455 in the Alamofire GitHub repo introduces the new Progress APIs and might be of help as well.
Solution 2
Alamofire 5, Swift 5
AF.download(urlString)
.downloadProgress { progress in
print("Download Progress: \(progress.fractionCompleted)")
}}
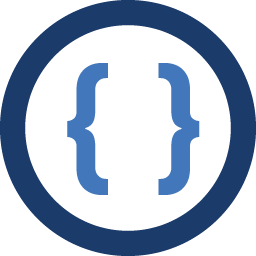
Admin
Updated on June 08, 2022Comments
-
Admin almost 2 years
I'm currently working on an iOS project which requires me to download 10 different files at once. I know the file size and the size of all the files combined but I'm struggling to find a way to calculate the progress across all download tasks.
progress.totalUnitCount = object.size // The size of all the files combined for file in files { let destination: DownloadRequest.DownloadFileDestination = { _, _ in let path = NSSearchPathForDirectoriesInDomains(FileManager.SearchPathDirectory.applicationSupportDirectory, FileManager.SearchPathDomainMask.userDomainMask, true) let documentDirectoryPath: String = path[0] let destinationURLForFile = URL(fileURLWithPath: documentDirectoryPath) return (destinationURLForFile, [.removePreviousFile, .createIntermediateDirectories]) } Alamofire.download(file.urlOnServer, to: destination) .downloadProgress(queue: .main, closure: { progress in }) .response { response in if let error = response.error { print(error) } } }
Most of this code is just for context.
I discovered, that up to Alamofire 3 there was this call:
.progress { bytesRead, totalBytesRead, totalBytesExpectedToRead in print("Bytes: \(bytesRead), Total Bytes: \(totalBytesRead), Total Bytes Expected: \(totalBytesExpectedToRead)") }
This is not present anymore and I'm wondering how I can get the same functionality.
Thank you in advance!