How to get the index number on scroll for listview in flutter?
There is no such easy way to do this, but you can use VisibilityDetector
from visibility_detector package:
You can get the index of the last list item that is completely visible on screen by wrapping each list item with a VisibilityDetector
.
itemBuilder: (context, index) {
return VisibilityDetector(
key: Key(index.toString()),
onVisibilityChanged: (VisibilityInfo info) {
if (info.visibleFraction == 1)
setState(() {
_currentItem = index;
print(_currentItem);
});
},
child: Post(index: index, title: 'Test', imageUrl: 'https://www.google.com',)
);
},
_currentItem
is a variable in the main widget's state that stores the index of the last visible item:
int _currentItem = 0;
Note: This is based on scroll direction, i.e., the last visible item's index equals to the index of the last item that is visible after the scroll; if the scroll is in forward direction, it is the index of the item on bottom of the screen, but if the scroll is in reverse direction, it is the index of the item on top of the screen. This logic can be easily changed though (e.g., using a queue to store the total visible items).
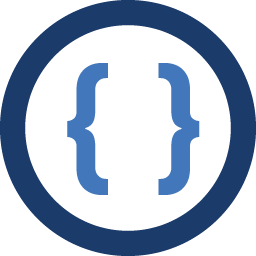
Admin
Updated on December 18, 2022Comments
-
Admin over 1 year
I am using below code for the listview builder in flutter application I need to get the index of the item in the list upon scrolling. Just Like function of
onPageChanged:(){}
while usingPageView.Builder
return ListView.builder( itemCount: posts.length, itemBuilder: (context, index) { final item = posts[index]; return Post(index: index, title: 'Test', imageUrl: 'https://www.google.com',); }, );
How can I get that?