How to get the innerHTML of selectable jquery element?
166,209
Solution 1
Try
.text() or .html() instead of .innerHTML
Solution 2
Use .val()
instead of .innerHTML
for getting value of selected option
Use .text()
for getting text of selected option
Thanks for correcting :)
Solution 3
$(function() {
$("#select-image").selectable({
selected: function( event, ui ) {
var $variable = $('.ui-selected').html();
console.log($variable);
}
});
});
or
$(function() {
$("#select-image").selectable({
selected: function( event, ui ) {
var $variable = $('.ui-selected').text();
console.log($variable);
}
});
});
or
$(function() {
$("#select-image").selectable({
selected: function( event, ui ) {
var $variable = $('.ui-selected').val();
console.log($variable);
}
});
});
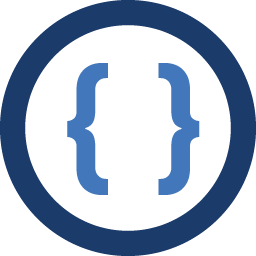
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have a php generated list whose list items are selectable using jquery selectable widget. The list for all intents and purposes is:
<ul id="#select-image"> <li class="ui-widget-content">Item 1</li> <li class="ui-widget-content">Item 2</li> <li class="ui-widget-content">Item 3</li> <li class="ui-widget-content">Item 4</li> <li class="ui-widget-content">Item 5</li> <li class="ui-widget-content">Item 6</li> <li class="ui-widget-content">Item 7</li> </ul>
And the jQuery selectable is declared as:
<script> $(function() { $("#select-image").selectable({ selected: function( event, ui ) { var $variable = $('.ui-selected').innerHTML; console.log($variable); } }); }); </script>
An event takes place after a list item has been selected, in the example it outputs to the browser console. The output however is "undefined." The selector
$('.ui-selected').
is correct as it shows as an object in the browser's console. Where am I going wrong?