How to get the owner and group of a folder with Python on a Linux machine?
25,588
Solution 1
Use os.stat()
to get the uid and gid of the file. Then, use pwd.getpwuid()
and grp.getgrgid()
to get the user and group names respectively.
import grp
import pwd
import os
stat_info = os.stat('/path')
uid = stat_info.st_uid
gid = stat_info.st_gid
print uid, gid
user = pwd.getpwuid(uid)[0]
group = grp.getgrgid(gid)[0]
print user, group
Solution 2
Since Python 3.4.4, the Path
class of pathlib
module provides a nice syntax for this:
from pathlib import Path
whatever = Path("relative/or/absolute/path/to_whatever")
if whatever.exists():
print("Owner: %s" % whatever.owner())
print("Group: %s" % whatever.group())
Solution 3
Use os.stat:
>>> s = os.stat('.')
>>> s.st_uid
1000
>>> s.st_gid
1000
st_uid
is the user id of the owner, st_gid
is the group id. See the linked documentation for other information that can be acuired through stat
.
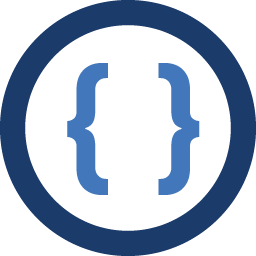
Author by
Admin
Updated on March 25, 2021Comments
-
Admin over 3 years
How can I get the owner and group IDs of a directory using Python under Linux?