How to get the root path of the project
Solution 1
os.Getwd()
does not return your source file path. It returns program current working directory (Usually the directory that you executed your program).
Example:
Assume I have a main.go in /Users/Arman/go/src/github.com/rmaan/project/main.go
that just outputs os.Getwd()
$ cd /Users/Arman/go/src/github.com/rmaan/project/
$ go run main.go
/Users/Arman/go/src/github.com/rmaan/project <nil>
If I change to other directory and execute there, result will change.
$ cd /Users/Arman/
$ go run ./go/src/github.com/rmaan/project/main.go
/Users/Arman <nil>
IMO you should explicitly pass the path you want to your program instead of trying to infer it from context (As it may change specially in production environment). Here is an example with flag
package.
package main
import (
"fmt"
"flag"
)
func main() {
var myPath string
flag.StringVar(&myPath, "my-path", "/", "Provide project path as an absolute path")
flag.Parse()
fmt.Printf("provided path was %s\n", myPath)
}
then execute your program as follows:
$ cd /Users/Arman/go/src/github.com/rmaan/project/
$ go run main.go --my-path /Users/Arman/go/src/github.com/rmaan/project/
provided path was /Users/Arman/go/src/github.com/rmaan/project/
$ # or more easily
$ go run main.go --my-path `pwd`
provided path was /Users/Arman/go/src/github.com/rmaan/project/
Solution 2
If your project is a git repository you can also use the git rev-parse
command with the --show-toplevel
:
cmdOut, err := exec.Command("git", "rev-parse", "--show-toplevel").Output()
if err != nil {
assert.Fail(t, fmt.Sprintf(`Error on getting the go-kit base path: %s - %s`, err.Error(), string(cmdOut)))
return
}
fmt.Println(strings.TrimSpace(string(cmdOut)))
It'll print out the git project home path wherever in the sub path you are
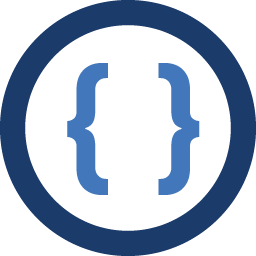
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I run the following code
workingDir, _ := os.Getwd()
inserver.go
file which is under the root project and get the root path, Now I need to move theserver.go
file to the following structuremyapp src server.go
and I want to ge the path of
go/src/myapp
currently after I move theserver.go
undermyapp->src>server.go
I got path ofgo/src/myapp/src/server.go
and I want to get the previews path ,How should I do it in go automatically? or should I put
../
explicit in get path ?