How to Get the Title of a HTML Page Displayed in UIWebView?
Solution 1
For those who just scroll down to find the answer:
- (void)webViewDidFinishLoad:(UIWebView *)webView{
NSString *theTitle=[webView stringByEvaluatingJavaScriptFromString:@"document.title"];
}
This will always work as there is no way to turn off Javascript in UIWebView.
Solution 2
WKWebView
has 'title' property, just do it like this,
func webView(_ wv: WKWebView, didFinish navigation: WKNavigation!) {
title = wv.title
}
I don't think UIWebView
is suitable right now.
Solution 3
If Javascript Enabled Use this :-
NSString *theTitle=[webViewstringByEvaluatingJavaScriptFromString:@"document.title"];
If Javascript Disabled Use this :-
NSString * htmlCode = [NSString stringWithContentsOfURL:[NSURL URLWithString:@"http://www.appcoda.com"] encoding:NSASCIIStringEncoding error:nil];
NSString * start = @"<title>";
NSRange range1 = [htmlCode rangeOfString:start];
NSString * end = @"</title>";
NSRange range2 = [htmlCode rangeOfString:end];
NSString * subString = [htmlCode substringWithRange:NSMakeRange(range1.location + 7, range2.location - range1.location - 7)];
NSLog(@"substring is %@",subString);
I Used +7 and -7 in NSMakeRange to eliminate the length of <title>
i.e 7
Solution 4
Edit: just saw you found out the answer... sheeeiiitttt
I literally just learned this! To do this, you don't even need to have it displayed in UIWebView. (But as you are using it, you can just get the URL of the current page)
Anyways, here's the code and some (feeble) explanation:
//create a URL which for the site you want to get the info from.. just replace google with whatever you want
NSURL *currentURL = [NSURL URLWithString:@"http://www.google.com"];
//for any exceptions/errors
NSError *error;
//converts the url html to a string
NSString *htmlCode = [NSString stringWithContentsOfURL:currentURL encoding:NSASCIIStringEncoding error:&error];
So we have the HTML code, now how do we get the title? Well, in every html-based doc the title is signaled by This Is the Title So probably the easiest thing to do is to search that htmlCode string for , and for , and substring it so we get the stuff in between.
//so let's create two strings that are our starting and ending signs
NSString *startPoint = @"<title>";
NSString *endPoint = @"</title>";
//now in substringing in obj-c they're mostly based off of ranges, so we need to make some ranges
NSRange startRange = [htmlCode rangeOfString:startPoint];
NSRange endRange = [htmlCode rangeOfString:endPoint];
//so what this is doing is it is finding the location in the html code and turning it
//into two ints: the location and the length of the string
//once we have this, we can do the substringing!
//so just for easiness, let's make another string to have the title in
NSString *docTitle = [htmlString substringWithRange:NSMakeRange(startRange.location + startRange.length, endRange.location)];
NSLog(@"%@", docTitle);
//just to print it out and see it's right
And that's really it! So basically to explain all the shenanigans going on in the docTitle, if we made a range just by saying NSMakeRange(startRange.location, endRange.location) we would get the title AND the text of startString (which is ) because the location is by the first character of the string. So in order to offset that, we just added the length of the string
Now keep in mind this code is not tested.. if there are any problems it might be a spelling error, or that I didn't/did add a pointer when i wasn't supposed to.
If the title is a little weird and not completely right, try messing around with the NSMakeRange-- I mean like add/subtract different lengths/locations of the strings --- anything that seems logical.
If you have any questions or there are any problems, feel free to ask. This my first answer on this website so sorry if it's a little disorganized
Solution 5
Here is Swift 4 version, based on answer at here
func webViewDidFinishLoad(_ webView: UIWebView) {
let theTitle = webView.stringByEvaluatingJavaScript(from: "document.title")
}
Related videos on Youtube
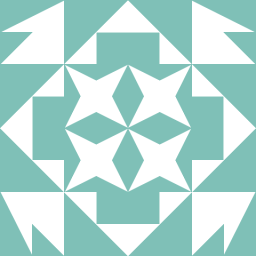
Comments
-
TechZen 6 months
I need to extract the contents of the title tag from an HTML page displayed in a UIWebView. What is the most robust means of doing so?
I know I can do:
- (void)webViewDidFinishLoad:(UIWebView *)webView{ NSString *theTitle=[webView stringByEvaluatingJavaScriptFromString:@"document.title"]; }
However, that only works if javascript is enabled.
Alternatively, I could just scan the text of the HTML code for the title but that feels a bit cumbersome and might prove fragile if the page's authors got freaky with their code. If it comes to that, what's the best method to use for processing the html text within the iPhone API?
I feel that I've forgotten something obvious. Is there a better method than these two choices?
Update:
Following from the answer to this question: UIWebView: Can You Disable Javascript? there appears to be no way to turn off Javascript in UIWebView. Therefore the Javascript method above will always work.
-
Dave DeLong almost 13 years+1 I also had to resort to the @"document.title" method.
-
margusholland over 11 yearsI was just searching for this and had scary visions of parsing the HTML. Very clever solution.
-
fishinear over 9 yearsSee also the following answer to a similar SO question: stackoverflow.com/a/2313430/908621
-
Kamil Wozniak about 9 yearsThere are many links in this portal, like: stackoverflow.com/questions/11704560/… or stackoverflow.com/questions/2301468/…
-
Daniel about 9 yearsThe Apple support community also has the same answer
-
-
Xiao almost 9 yearsusing this, if the encoding of the webpage is not 'utf-8', the title would be messy.
-
TechZen over 7 yearsCan you disable Javascript in UIWebview now? Back in 2010 you couldn't.
-
Pawandeep Singh over 7 yearsI don't know about IOS prior to IOS 8 but you can do it, Go To Settings --> Safari --> Advanced --> Javascript On/Off
-
Eddie about 7 yearsHow do you know if javascript is enable or disable in the code?
-
Jayprakash Dubey over 4 yearsHow about Swift 4?