How to get the values of a ConfigurationSection of type NameValueSectionHandler
Solution 1
Suffered from exact issue. Problem was because of NameValueSectionHandler in .config file. You should use AppSettingsSection instead:
<configuration>
<configSections>
<section name="DEV" type="System.Configuration.AppSettingsSection" />
<section name="TEST" type="System.Configuration.AppSettingsSection" />
</configSections>
<TEST>
<add key="key" value="value1" />
</TEST>
<DEV>
<add key="key" value="value2" />
</DEV>
</configuration>
then in C# code:
AppSettingsSection section = (AppSettingsSection)ConfigurationManager.GetSection("TEST");
btw NameValueSectionHandler is not supported any more in 2.0.
Solution 2
Here's a good post that shows how to do it.
If you want to read the values from a file other than the app.config, you need to load it into the ConfigurationManager.
Try this method: ConfigurationManager.OpenMappedExeConfiguration()
There's an example of how to use it in the MSDN article.
Solution 3
Try using an AppSettingsSection
instead of a NameValueCollection
. Something like this:
var section = (AppSettingsSection)config.GetSection(sectionName);
string results = section.Settings[key].Value;
Solution 4
The only way I can get this to work is to manually instantiate the section handler type, pass the raw XML to it, and cast the resulting object.
Seems pretty inefficient, but there you go.
I wrote an extension method to encapsulate this:
public static class ConfigurationSectionExtensions
{
public static T GetAs<T>(this ConfigurationSection section)
{
var sectionInformation = section.SectionInformation;
var sectionHandlerType = Type.GetType(sectionInformation.Type);
if (sectionHandlerType == null)
{
throw new InvalidOperationException(string.Format("Unable to find section handler type '{0}'.", sectionInformation.Type));
}
IConfigurationSectionHandler sectionHandler;
try
{
sectionHandler = (IConfigurationSectionHandler)Activator.CreateInstance(sectionHandlerType);
}
catch (InvalidCastException ex)
{
throw new InvalidOperationException(string.Format("Section handler type '{0}' does not implement IConfigurationSectionHandler.", sectionInformation.Type), ex);
}
var rawXml = sectionInformation.GetRawXml();
if (rawXml == null)
{
return default(T);
}
var xmlDocument = new XmlDocument();
xmlDocument.LoadXml(rawXml);
return (T)sectionHandler.Create(null, null, xmlDocument.DocumentElement);
}
}
The way you would call it in your example is:
var map = new ExeConfigurationFileMap
{
ExeConfigFilename = @"c:\\foo.config"
};
var configuration = ConfigurationManager.OpenMappedExeConfiguration(map, ConfigurationUserLevel.None);
var myParamsSection = configuration.GetSection("MyParams");
var myParamsCollection = myParamsSection.GetAs<NameValueCollection>();
Solution 5
This is an old question, but I use the following class to do the job. It's based on Scott Dorman's blog:
public class NameValueCollectionConfigurationSection : ConfigurationSection
{
private const string COLLECTION_PROP_NAME = "";
public IEnumerable<KeyValuePair<string, string>> GetNameValueItems()
{
foreach ( string key in this.ConfigurationCollection.AllKeys )
{
NameValueConfigurationElement confElement = this.ConfigurationCollection[key];
yield return new KeyValuePair<string, string>
(confElement.Name, confElement.Value);
}
}
[ConfigurationProperty(COLLECTION_PROP_NAME, IsDefaultCollection = true)]
protected NameValueConfigurationCollection ConfCollection
{
get
{
return (NameValueConfigurationCollection) base[COLLECTION_PROP_NAME];
}
}
The usage is straightforward:
Configuration configuration = ConfigurationManager.OpenExeConfiguration(ConfigurationUserLevel.None);
NameValueCollectionConfigurationSection config =
(NameValueCollectionConfigurationSection) configuration.GetSection("MyParams");
NameValueCollection myParamsCollection = new NameValueCollection();
config.GetNameValueItems().ToList().ForEach(kvp => myParamsCollection.Add(kvp));
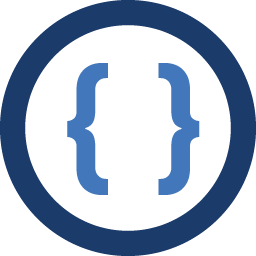
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I'm working with C#, Framework 3.5 (VS 2008).
I'm using the
ConfigurationManager
to load a config (not the default app.config file) into a Configuration object.Using the Configuration class, I was able to get a
ConfigurationSection
, but I could not find a way to get the values of that section.In the config, the
ConfigurationSection
is of typeSystem.Configuration.NameValueSectionHandler
.For what it worth, when I used the method
GetSection
of theConfigurationManager
(works only when it was on my default app.config file), I received an object type, that I could cast into collection of pairs of key-value, and I just received the value like a Dictionary. I could not do such cast when I receivedConfigurationSection
class from the Configuration class however.EDIT: Example of the config file:
<configuration> <configSections> <section name="MyParams" type="System.Configuration.NameValueSectionHandler" /> </configSections> <MyParams> <add key="FirstParam" value="One"/> <add key="SecondParam" value="Two"/> </MyParams> </configuration>
Example of the way i was able to use it when it was on app.config (the "GetSection" method is for the default app.config only):
NameValueCollection myParamsCollection = (NameValueCollection)ConfigurationManager.GetSection("MyParams"); Console.WriteLine(myParamsCollection["FirstParam"]); Console.WriteLine(myParamsCollection["SecondParam"]);
-
Admin over 13 yearsAs i said in my post, This is what i did. I received Configuraion class, and from it i received a ConfigurationSection. My question was how can i get the values from that ConfigurationSection object? i didn't ask how to get the Configuration object.. Thanks anyway!
-
Admin over 13 yearsby the way, in the example you gave me they are not using the Configuration class that comes from OpenMappedExeConfiguration, but they are using the default app.config (which can be used using the .GetSection/.GetConfig methods, but as i said in my post- i did that already. but its no good for me since its good for app.config only). and for the msdn, i could not find the answer to my question there..
-
MonkeyWrench over 13 yearsOk, the more I look into it, the more I see it isn't easy to do. The basic problem is that you're trying to mix .Net 1.1 configuration style code and 2.0. Read this, it'll point you in the right direction: eggheadcafe.com/software/aspnet/30832856/…
-
nerijus about 11 yearsnope this way you will get "Unable to cast object of type 'System.Configuration.DefaultSection' to type 'System.Configuration.AppSettingsSection'."
-
h9uest about 11 years@nerijus : come on man ... Of course one of Steven's assumptions is that you actually change the type of the section accordingly ... No offense, but you really could think before commenting. I tried this solution and it works. +1 for Steven
-
Peter Ivan over 10 years@h9uest: It's not so straightforward, as Liran wrote the section is
System.Configuration.NameValueSectionHandler
. This answer seems more like a kind-of-dirty hack. -
Jone Polvora over 9 yearsCasting to AppSettings section didn't worked for me. Instead, i've casted to NameValueCollection and worked.
-
Hari over 9 years@MonkeyWrench The page is no more available.
-
MonkeyWrench over 9 yearsLooks like bluefeet fixed that. Thanks.
-
MonkeyWrench about 9 yearsAw... why the downvote? I can't fix the internet. The link is 5 years old. The MSDN has a good example as well.
-
Liam almost 9 yearsThis answer appears to be suffering from link rot, this is why link only answers are discouraged.
-
RayLoveless over 7 yearsGreat blog post. Thanks for sharing.
-
Joseph almost 6 yearsIt's so nice to see somebody answering the question asked. I have been digging through reams of answers to questions about handling OpenExeConfiguration GetSection with "answers" that redirect people to ConfigurationManager.GetSection which doesn't so the same thing.
-
Onsokumaru over 5 yearsDownvote: It's a good answer in general about the config topic for the beginner/standard usecase, but it's a useless answer for the specific problem of the initial question. I ran into the same problem as Liran B and the blogpost helps absolutely not. The question is how to deal with the
ConfigurationSection
-object. -
Onsokumaru over 5 yearsI had a little bit trouble to get my section working (some mistakes while loading the config file itself), but after that this solution worked perfectly. Now I can split my config file in multiple sections and easily access the values via AppSettingsSection.
-
nivs1978 almost 4 yearsYou should be able to just call the Get method: var myParamsCollection = myParamsSection.Get<NameValueCollection>();
-
aj go over 2 yearsnot working on my side
-
wavydavy about 2 yearsWhen I try the above I'm getting "Unable to find section handler type..." error. In your example what does the XML look like? I'm using the OP XML "<section name="MyParams" type="System.Configuration.NameValueSectionHandler" />"