How to get Unix timestamp using Bootstrap-datetimepicker
Solution 1
The eonasdan datetimepicker has a good API that you can use to programmatically interact with the picker.
You can use the date()
method that:
Returns the component's model current date, a
moment
object ornull
if not set.
Since the value return by date()
is a moment object you can use unix()
to get Unix timestamp (the number of seconds since the Unix Epoch).
Here a working sample:
function getValue() {
var date = $('#datetimepicker1').data("DateTimePicker").date();
if( date ){
alert(date.unix());
}
}
$('#datetimepicker1').datetimepicker();
<link href="//cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.css" rel="stylesheet"/>
<link href="//cdnjs.cloudflare.com/ajax/libs/bootstrap-datetimepicker/4.17.47/css/bootstrap-datetimepicker.css" rel="stylesheet"/>
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.js"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/moment.js/2.18.1/moment.min.js"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/js/bootstrap.js"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/bootstrap-datetimepicker/4.17.47/js/bootstrap-datetimepicker.min.js"></script>
<div class='input-group date' id='datetimepicker1'>
<input type='text' class="form-control" />
<span class="input-group-addon">
<span class="glyphicon glyphicon-calendar"></span>
</span>
</div>
<button onclick="getValue()" class="btn btn-primary">Get value</button>
Remember that, as the docs says:
Note All functions are accessed via the
data
attribute e.g.$('#datetimepicker').data("DateTimePicker").FUNCTION()
Solution 2
Short Answer: since that plugin work together with moment.js you can use the momentjs method
unix()
, like in the following way:
moment($('#datetimepicker1').data('date')).unix()
Update(I was testing on Internet Explorer 11)
$('#datetimepicker1').data('DateTimePicker').date().unix()
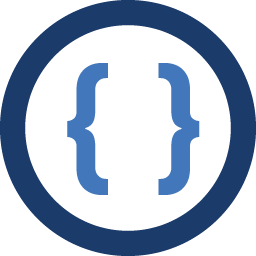
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I am using 3rd party date time picker from https://eonasdan.github.io/bootstrap-datetimepicker/
It is working fine, however, I'd like to get the value in Unix timestamp format. How do I do that? There is no formatting option for Unix. https://eonasdan.github.io/bootstrap-datetimepicker/Functions/#format
function getValue() { var date = $('#datetimepicker1').data('date'); alert(date); // shows 05/25/2017 5:43 PM } <div class='input-group date' id='datetimepicker1'> <input type='text' class="form-control" /> <span class="input-group-addon"> <span class="glyphicon glyphicon-calendar"></span> </span> </div>
-
Admin about 7 yearsthe answer i was looking for
-
Admin about 7 yearsthis actually gives the browser deprecation warning for moment.js as seen here (stackoverflow.com/questions/39969570/…)
-
VincenzoC about 7 yearsYou are getting Deprecation Warning because
$('#datetimepicker1').data('date')
returns a string that is not in recognized format. This is not the right approach to get picker value, use thedate()
method of the API instead.