How to get Usage Access Permission programmatically
You can simply give this Permission on the manifest, ignoring just this permission error:
<uses-permission
android:name="android.permission.PACKAGE_USAGE_STATS"
tools:ignore="ProtectedPermissions"/>
Than use Following code for permission
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (!isAccessGranted()) {
Intent intent = new Intent(Settings.ACTION_USAGE_ACCESS_SETTINGS);
startActivity(intent);
}
}
private boolean isAccessGranted() {
try {
PackageManager packageManager = getPackageManager();
ApplicationInfo applicationInfo = packageManager.getApplicationInfo(getPackageName(), 0);
AppOpsManager appOpsManager = (AppOpsManager) getSystemService(Context.APP_OPS_SERVICE);
int mode = 0;
if (android.os.Build.VERSION.SDK_INT > android.os.Build.VERSION_CODES.KITKAT) {
mode = appOpsManager.checkOpNoThrow(AppOpsManager.OPSTR_GET_USAGE_STATS,
applicationInfo.uid, applicationInfo.packageName);
}
return (mode == AppOpsManager.MODE_ALLOWED);
} catch (PackageManager.NameNotFoundException e) {
return false;
}
}
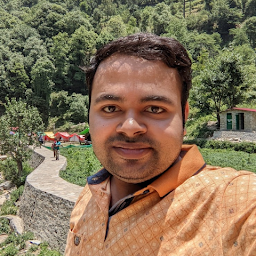
Vivek Mishra
Collaborative Mobile Developer with a reputation for building lasting relationships among team members, partnering across departments to produce highly effective content that meets desired outcomes. App-oriented architect with meticulous attention to detail in strategic design and problem-solving.
Updated on June 18, 2022Comments
-
Vivek Mishra about 2 years
My app needs to have Usage Access Permission in order to get information about the current running app on user's phone. I am able to successfully implement it using the following code with the help from the following link.
Here is my working code
public void showDialog() { if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.LOLLIPOP) { @SuppressWarnings("WrongConstant") UsageStatsManager usm = (UsageStatsManager) getSystemService("usagestats"); long time = System.currentTimeMillis(); List<UsageStats> appList = usm.queryUsageStats(UsageStatsManager.INTERVAL_DAILY, time - 1000 * 1000, time); if (appList.size()==0) { AlertDialog alertDialog = new AlertDialog.Builder(this) .setTitle("Usage Access") .setMessage("App will not run without usage access permissions.") .setPositiveButton("Settings", new DialogInterface.OnClickListener() { @TargetApi(Build.VERSION_CODES.LOLLIPOP) public void onClick(DialogInterface dialog, int which) { // continue with delete Intent intent = new Intent(Settings.ACTION_USAGE_ACCESS_SETTINGS); // intent.setComponent(new ComponentName("com.android.settings","com.android.settings.Settings$SecuritySettingsActivity")); intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); startActivityForResult(intent,0); } }) .setNegativeButton("Cancel", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { // do nothing dialog.dismiss(); } }) .setIcon(android.R.drawable.ic_dialog_alert) .create(); alertDialog.getWindow().setType(WindowManager.LayoutParams.TYPE_SYSTEM_ALERT); alertDialog.show(); } else { Intent intent = new Intent(this, PackageService.class); startService(intent); finish(); } }else { Intent intent = new Intent(this, PackageService.class); startService(intent); finish(); } }
This code will show a user an alert dialog that Usage Access permission is required and will take user to the settings screen from where it can be enabled.
Everything works perfectly with this. Only thing that I want is that how to give my app usage access permission by default without showing any dialog to user until he/she doesn't turn it off manually?
-
kash almost 7 years@samchaudhari with the given method, the user still has to open the Usage access page and turn it on manually. Does it turn it on automatically for you? without any user input?
-
sam chaudhari almost 7 years@kash there is not any way to turn it on automatically. user must turn it on manually.
-
Ahmad Joyia over 5 yearshow we add tutorial screen in usage setting screen