How to get user id from email address in Microsoft Graph API C#
Solution 1
You can look up a user a few different ways.
From the /users
endpoint you can either use their id
(the GUID assigned to each account) or their userPrincipalName
(their email alias for the default domain):
// Retrieve a user by id
var user = await graphClient
.Users["00000000-0000-0000-0000-000000000000"]
.Request()
.GetAsync();
// Retrieve a user by userPrincipalName
var user = await graphClient
.Users["[email protected]"]
.Request()
.GetAsync();
If you're using either the Authorization Code or Implicit OAuth grants, you can also look up the user who authenticated via the /me
endpoint:
var user = await graphClient
.Me
.Request()
.GetAsync();
Solution 2
Besides these correct answers above.
userPrincipalName
or id
is not the external email address. That was my case when registration is done manually. You can use this filter:
var user = await _graphClient.Users
.Request()
.Filter($"identities/any(c:c/issuerAssignedId eq '{email}' and c/issuer eq 'contoso.onmicrosoft.com')")
.GetAsync();
src: https://docs.microsoft.com/en-us/graph/api/user-list?view=graph-rest-1.0&tabs=csharp
Using this configuration to create an user:
Microsoft.Graph.User graphUser = new Microsoft.Graph.User
{
AccountEnabled = true,
PasswordPolicies = "DisablePasswordExpiration,DisableStrongPassword",
PasswordProfile = new PasswordProfile
{
ForceChangePasswordNextSignIn = false,
Password = accountModel.Password,
},
Identities = new List<ObjectIdentity>
{
new ObjectIdentity()
{
SignInType = "emailAddress",
Issuer ="contoso.onmicrosoft.com",
IssuerAssignedId = email
}
},
};
Solution 3
You can retrieve user's details from Graph API using id
or userPrincipalName
(which is an email address).
From Microsoft Graph API reference:
GET /users/{id | userPrincipalName}
Have you tried to use the email address as objectID
?
Solution 4
If you need retrieve a guest user may use:
public static async Task<User> GetGuestUserByEmail(string email)
{
try
{
var request = await graphClient
.Users
.Request()
.Filter($"userType eq 'guest' and mail eq '{email}'") // apply filter
.GetAsync();
var guestUsers = request.CurrentPage.ToList();
var user = guestUsers.FirstOrDefault();
return user;
}
catch (ServiceException ex)
{
Console.WriteLine($"Error: {ex.Message}");
return null;
}
}
Solution 5
There actually is no truly reliable way to do this. Email addresses in AAD are not unique. The UPN is often an email address, and it is unique, but there's a nasty catch: If the person happens to have a long email address AND they're a guest user, the UPN appears to be the email truncated to 54 characters, then have #EXT# tacked on, and it can even be changed after the fact to have the #EXT# removed where it can be up to 64 characters before the @ symbol, and doesn't have to be anything resembling their actual email address.
The only way you're supposed to actually be able to find them is with their user object's GUID.
https://docs.microsoft.com/en-us/answers/questions/81053/user-principal-name-and-ext.html
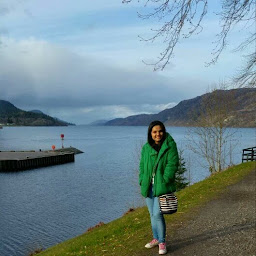
sidi shah
Updated on July 09, 2022Comments
-
sidi shah almost 2 years
I want to add User to a Group but I don't have the User's
id
, I only have the email address.Here is the code:
User userToAdd = await graphClient .Users["objectID"] .Request() .GetAsync(); await graphClient .Groups["groupObjectID"] .Members .References .Request() .AddAsync(userToAdd);
Can someone help how do I retrieve ObjectId (User ID) from an email address using Microsoft Graph?
-
Tassisto almost 3 yearsIs there a way to filter by User Principal Name?
-
Marc LaFleur almost 3 yearsA User Principal Name is unique to a tenant so filtering by UPN would produce a collection with a single item. That item would be the same as
.Users["[email protected]"]
. -
Pieter Heemeryck over 2 yearsThis is not what OP asked. The email address can be different from the UPN and need not be unique unfortunately.
-
Pieter Heemeryck over 2 yearsOP is asking for email, not UPN or id. Email need not be the same as the UPN..