How to handle jdbc.queryForObject if it doesn't return a row
Solution 1
Try this:
return DataAccessUtils.singleResult(jdbcTemplate.query(sql, new SingleColumnRowMapper<Boolean>(), param1, param2));
Solution 2
queryForObject method of JdbcTemplate expects that your query should return one row always else it will throw EmptyResultDataAccessException. You can simply use query method with ResultSetExtractor instead of queryForObject. ResultSetExtractor - extractData(ResultSet rs) method would return an arbitrary result object, or null if no data returned. You can throw your relevant exception if it returns null.
return jdbc.query(sql, new ResultSetExtractor<Boolean>() {
@Override
public Boolean extractData(ResultSet rs) throws SQLException,
DataAccessException {
return rs.next() ? rs.getBoolean("column_name") : null;
}
});
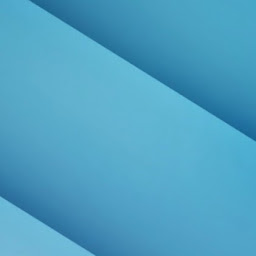
Oleg
Updated on June 10, 2022Comments
-
Oleg almost 2 years
I would like to know how to properly use jdbc in my case.
The
saveLinkHistory
column is a bit(1) type in mysql.public boolean getIsSavedLinkHistory(String name) { String sql = "select saveLinkHistory from users where name = ?"; Boolean isTracked = jdbcTemplateObject.queryForObject(sql, new Object[] { name }, Boolean.class); return isTracked; }
The query worked well until I got an error of
Incorrect result size: expected 1, actual 0
because sometimes thename
didn't exist, thequeryForObject
method expects that I ALWAYS get 1 row as a result.How can I handle this case, just throw an exception that says "name" doesn't exist ? and by the way, is
Boolean
ok here ? because I didn't see such code before.