How to have localStorage value of true?
10,738
I was wondering if its possible for localStorage to have a Boolean value instead of a string?
No, web storage only stores strings. To store more rich data, people typically use JSON and stringify when storing and parse when retrieving.
Storing:
var test = true;
localStorage.setItem("test", JSON.stringify(test));
Retrieving:
test = JSON.parse(localStorage.getItem("test"));
console.log(typeof test); // "boolean"
You don't need JSON for just a boolean, though; you could just use ""
for false and any other string for true, since ""
is a "falsey" value (a value that coerces to false when treated as a boolean).
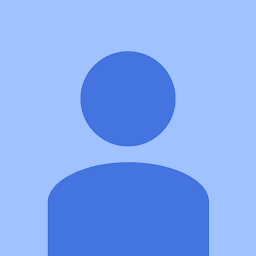
Author by
GoogleFailedMe
Updated on July 22, 2022Comments
-
GoogleFailedMe almost 2 years
I was wondering if its possible for localStorage to have a Boolean value instead of a string?
Using JS only no JSON if its impossible or can be done in JS a different way please let me know thanks
http://jsbin.com/qiratuloqa/1/
//How to set localStorage "test" to true? test = localStorage.getItem("test"); localStorage.setItem("test", true); if (test === true) { alert("works"); } else { alert("Broken"); } /* String works fine. test = localStorage.getItem("test"); localStorage.setItem("test", "hello"); if (test === "hello") { alert("works"); } else { alert("Broken"); } */