How to have Python check if a file exists and create it if it doesn't?
23,025
Solution 1
This is the best way:
try:
with open(filename) as file:
# do whatever
except IOError:
# generate the file
There's also os.path.exists(), but this can be a security concern.
Solution 2
This one-liner will check to see if the file exists, and create it if it doesn't.
open("KEEP-IMPORTANT.txt", "a")
Solution 3
import os.path
from os import path
if path.exists('highScore.txt'):
print("It exists")
else:
print("It doesn't exist")
Solution 4
Another clean and small solution if you want to check and create the file only with out writing anything right away would be this.
import os
if os.path.exists(file_path) == False:
open(file_path, "w").close
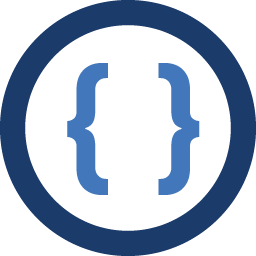
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
How to have Python look and see if there is a file it needs and if there isn't create one?
Basically I want Python to look for my file name
KEEP-IMPORTANT.txt
, but when I create my app usingpy2app
it doesn't work because it doesn't have the file. When I try and make the file it won't work (I think because python has to generate it).I want Python to check if the file exists so that if it does then it doesn't generate the file, otherwise it does.
-
Octipi about 11 yearsI contrived an example to test this method for flaws. In python 2.7 permission errors raise IOError. I revoked read access for a test file but allowed write. Trying to read the file produced an IOError and I was able to overwrite it using this exception. This will not work in python 3 as reading the file produces PermissionError. This is probably a niche scenario that is unlikely to happen. I wonder what other ways IOError could occur with an existing file.
-
Alex Hammel about 11 yearsIf you don't have rw permissions for the file, won't trying to clobber it just raise another IOError?
-
Octipi about 11 yearsIn python 3 it would raise PermissionError. I tested the unlikely scenario that you had write permissions but not read permissions. In this case, for python 2.7, reading the file raised IOError but I could still write to it with write permissions.
-
cutrightjm over 9 years+1 for it making the file and no errors - but what about also deleting previous bits of the file?
-
Chris Johnson about 8 yearsThis answer introduces a race condition -- it's possible for the file to have been created by another process between the time you try to open it and the time you try to create it. The right answer is to open the file with the right options to control write, append, truncate etc.
-
D09r almost 6 years@cutrightjm Change the mode to "w" (write). Will overwrite the existing contents.