How to hide soft input keyboard on flutter after clicking outside TextField/anywhere on screen?
Solution 1
You are doing it in the wrong way, just try this simple method to hide the soft keyboard. you just need to wrap your whole screen in the GestureDetector
method and onTap
method write this code.
FocusScope.of(context).requestFocus(new FocusNode());
Here is the complete example:
new Scaffold(
body: new GestureDetector(
onTap: () {
FocusScope.of(context).requestFocus(new FocusNode());
},
child: new Container(
//rest of your code write here
)
)
Updated (May 2021)
return GestureDetector(
onTap: () => FocusManager.instance.primaryFocus?.unfocus(),
child: Scaffold(
appBar: AppBar(
title: Text('Login'),
),
body: Body(),
),
);
This will work even when you touch the AppBar, new
is optional in Dart 2. FocusManager.instance.primaryFocus
will return the node that currently has the primary focus in the widget tree.
Conditional access in Dart with null-safety
Solution 2
Updated
Starting May 2019, FocusNode
now has unfocus
method:
Cancels any outstanding requests for focus.
This method is safe to call regardless of whether this node has ever requested focus.
Use unfocus
if you have declared a FocusNode
for your text fields:
final focusNode = FocusNode();
// ...
focusNode.unfocus();
My original answer suggested detach
method - use it only if you need to get rid of your FocusNode
completely. If you plan to keep it around - use unfocus
instead.
If you have not declared a FocusNode
specifically - use unfocus
for the FocusScope
of your current context:
FocusScope.of(context).unfocus();
See revision history for the original answer.
Solution 3
Wrap whole screen in GestureDetector
as
new Scaffold(
body: new GestureDetector(
onTap: () {
// call this method here to hide soft keyboard
FocusScope.of(context).requestFocus(new FocusNode());
},
child: new Container(
-
-
-
)
)
Solution 4
I've added this line
behavior: HitTestBehavior.opaque,
to the GestureDetector and it seems to be working now as expected.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(AppLocalizations.of(context).calculatorAdvancedStageTitle),
),
body: GestureDetector(
behavior: HitTestBehavior.opaque,
onTap: () {
FocusScope.of(context).requestFocus(new FocusNode());
},
child: Padding(
padding: const EdgeInsets.only(
left: 14,
top: 8,
right: 14,
bottom: 8,
),
child: Text('Work'),
),
)
);
}
Solution 5
Just as a small side note:
If you use ListView
its keyboardDismissBehavior
property could be of interest:
ListView(
keyboardDismissBehavior: ScrollViewKeyboardDismissBehavior.onDrag,
children: [],
)
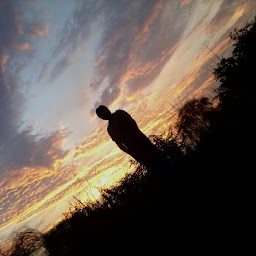
Comments
-
Ammy Kang almost 2 years
Currently, I know the method of hiding the soft keyboard using this code, by
onTap
methods of any widget.FocusScope.of(context).requestFocus(new FocusNode());
But I want to hide the soft keyboard by clicking outside of TextField or anywhere on the screen. Is there any method in
flutter
to do this?-
Günter Zöchbauer over 5 yearsYou can wrap your whole screen in a docs.flutter.io/flutter/widgets/GestureDetector-class.html and call above code in
onTap: () => FocusScope.of(context).requestFocus(new FocusNode());
-
Ammy Kang over 5 yearsThanks @GünterZöchbauer. is there any touch event method, as tapping is not gonna resolve my issue. Keyboard is hiding on onTap method. I need to hide the keyboard frequently as I touch the screen.
-
Günter Zöchbauer over 5 yearsSorry, I don't follow. why would tapping not solve your issue? You are tapping on the background or similar. When that happens, you call ...requestFocus...
-
Ammy Kang over 5 yearsI am working with TabBar and have search view box on each Tab screens. when I swipe from one tab to another then it doesn't swipe to another tab and comes back to same tab if keyboard is up on the screen or if there is text in TextField of SearchView. am having tab swiping issue mainly when keyboard is up otherwise tab swiping working fine.
-
Günter Zöchbauer over 5 yearsHow does that prevent you from applying my suggestion?
-
Ammy Kang over 5 yearskeyboard is hiding on onTap but still facing same issue, may be keyboard is hiding little late.
-
Günter Zöchbauer over 5 yearsI see. Sorry, don't know how to work around that.
-
boformer over 5 yearsTry to use a
Listener
widget for access to direct touch events. -
Ammy Kang over 5 yearsHow can I add
Listener
widget, can you please gimme an example? -
diegoveloper over 5 yearstry the package I created :) pub.dartlang.org/packages/keyboard_actions
-
-
AndrewS almost 5 yearsActually only with a
behavior: HitTestBehavior.translucent,
parameteronTap
is always called. It didn't work on some taps for me without this parameter. -
Daryl Wong almost 5 yearsSomehow the hittestbehaviour is needed for it to work in iOS.
-
Rodrigo Vieira about 4 years
detach
is no longer available, I believe. I tried calling it, but it does not work - it says there's no function with that name. -
Egor Zvonov about 4 years@RodrigoVieira thank you for pointing that out. I removed outdated information from my post.
-
Bagusflyer about 4 years
unfocus
is called but nothing happen. The current focus not lost. -
marikamitsos about 4 yearsIt would be more useful if you could also add here the main points of your linked site.
-
jdog almost 4 yearsThis version redraws your entire view heirarchy; whatever is inside the gestureDetector. I believe the issue is the currentFocus.unfocus() call. Use the selected answer above.
-
dbyuvaraj almost 4 yearsThis answer is outdated. Please check the updated answer for v1.17.1 (at least :)) here stackoverflow.com/a/61986983/705842
-
Avnish Nishad almost 4 yearsSometime gesture detector does not work on the whole screen then just wraps the scaffold inside GestureDetector Widget.
-
alireza easazade over 3 yearsmaybe try
onPanDown
instead ofonTap
or maybe another gesture -
Ali80 over 3 yearsyour small side note is big!
-
Fuad All over 3 yearsThanks ! awesome note. Perfect solution for Searchdelegate.
-
Dharaneshvar over 3 yearsI use Singlechildscrollview and if the empty spaces below are touched it doesn't work. Is there any solution for it?
-
Marcello Câmara over 3 years@Dharaneshvar the answer is: put your GestureDetecfor before Scaffold. Enjoy =)
-
Salim Murshed about 3 yearsWrite some details.
-
Ramshankar TV almost 3 yearsWrite this code in your main.dart inside build function.So that it will work for the whole application
-
Alexa289 almost 3 yearsGesture detector should be on Scaffold body directly, otherwise it won't work
-
alireza daryani almost 3 yearsYeah, this is best solution instead of remove focus.
-
ddisdevelpgelis over 2 yearsI defined this in the main function at the beginning ( void main() {) ). However, it doesn't work. Did I just make a mistake?
-
Hardik about 2 yearsonTapDown is better than onTap.
onTapDown: (_) => FocusManager.instance.primaryFocus?.unfocus()
-
icyNerd almost 2 yearswhere is it added? in GestureDetector?
-
icyNerd almost 2 yearsSame. Only this works for me. Thanks.
-
efesahin almost 2 yearsyeah, after a year, it works nicely on iOS...
-
SePröbläm almost 2 yearsJust for the records: Creating
FocusNode
s on demand without disposing them is likely to cause memory leaks...