How to hide ToolBar when I scrolling content up?
Solution 1
you have to do many changes in your both layout. first use CoordinatorLayout
in activity_main.XML
like below(change theme as per your requirement).
<?xml version="1.0" encoding="utf-8"?>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/main_content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true">
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"
app:layout_scrollFlags="scroll|enterAlways"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light" />
</android.support.design.widget.AppBarLayout>
<include layout="@layout/content_main" />
</android.support.design.widget.CoordinatorLayout>
in content_main.XML
use android.support.v4.widget.NestedScrollView
instead of ScrollView
.
also use app:layout_behavior="@string/appbar_scrolling_view_behavior"
inside android.support.v4.widget.NestedScrollView
like below.
<android.support.v4.widget.NestedScrollView
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:android="http://schemas.android.com/apk/res/android"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textone"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="hello world jheds sdjhs jds sjbs skjs ksjs kksjs ksj sdd dskd js sk "
android:textSize="25dp"
android:textStyle="bold" />
/// Add your other code here
</LinearLayout>
</android.support.v4.widget.NestedScrollView>
Solution 2
This is the best scenario to make use of CoordinatorLayout in your app. CoordinatorLayout is a super-powered FrameLayout which has got a lot of nifty animation tricks upon its sleeves.
The Design library introduces CoordinatorLayout, a layout which provides an additional level of control over touch events between child views, something which many of the components in the Design library take advantage of.
You can start with this and this tutorial.
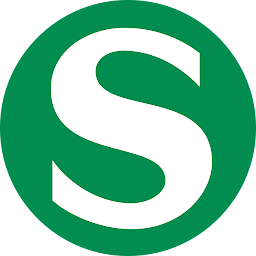
chanti
Updated on December 18, 2021Comments
-
chanti over 2 years
I am trying to hide my tool bar when I scroll my text and image with content. Here I use scrollView for getting scroll content. When I scroll content up, how to hide the tool bar?
Here is my XMl code:
content_main.XML:
<android.support.v4.widget.NestedScrollView xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" xmlns:android="http://schemas.android.com/apk/res/android" app:layout_behavior="@string/appbar_scrolling_view_behavior"> <LinearLayout android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <LinearLayout android:paddingTop="?android:attr/actionBarSize" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_marginLeft="10dp" android:layout_marginRight="10dp" android:id="@+id/textone" android:layout_width="match_parent" android:layout_height="match_parent" android:textSize="23dp" android:textStyle="bold" android:text="hello world jheds sdjhs jds sjbs skjs ksjs kksjs ksj sdd dskd js sk "/> <ImageView android:id="@+id/imge" android:layout_width="match_parent" android:layout_height="250dp" android:src="@drawable/imag_bg"/> <TextView android:id="@+id/texttwo" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_marginLeft="10dp" android:layout_marginRight="10dp" android:text="Pretty good, the Toolbar is moving along with the list and getting back just as we expect it to. This is thanks to the restrictions that we put on the mToolbarOffset variable. If we would omit checking if it’s bigger than 0 and lower than mToolbarHeight then when we would scroll up our list, the Toolbar would move along far away off the screen, so to show it back you would have to scroll the list down to 0. Right now it just scrolls up to mToolbarHeight position and not more so it’s “sitting” right above the list all of the time and if we start scrolling down, we can see it immediately showing. up our list, the Toolbar would move along far away off the screen, so to show it back you would have to scroll the list down to 0. Right now it just scrolls up to mToolbarHeight position and not more so it’s “sitting” right above the list all of the time and if we start scrolling down, we can see it immediately showing up our list, the Toolbar would move along far away off the screen, so to show it back you would have to scroll the list down to 0. Right now it just scrolls up to mToolbarHeight position and not more so it’s “sitting” right above the list all of the time and if we start scrolling down, we can see it immediately showing up our list, the Toolbar would move along far away off the screen, so to show it back you would have to scroll the list down to 0. Right now it just scrolls up to mToolbarHeight position and not more so it’s “sitting” right above the list all of the time and if we start scrolling down, we can see it immediately showing up our list, the Toolbar would move along far away off the screen, so to show it back you would have to scroll the list down to 0. Right now it just scrolls up to mToolbarHeight position and not more so it’s “sitting” right above the list all of the time and if we start scrolling down, we can see it immediately showing up our list, the Toolbar would move along far away off the screen, so to show it back you would have to scroll the list down to 0. Right now it just scrolls up to mToolbarHeight position and not more so it’s “sitting” right above the list all of the time and if we start scrolling down, we can see it immediately showing It works pretty well, but this is not what we want. It feels weird that you can stop it in the middle of the scroll and the Toolbar will stay half visible. Actually this is how it’s done in Google Play Games app which I consider as a bug It works pretty well, but this is not what we want. It feels weird that you can stop it in the middle of the scroll and the Toolbar will stay half visible. Actually this is how it’s done in Google Play Games app which I consider as a bug It works pretty well, but this is not what we want. It feels weird that you can stop it in the middle of the scroll and the Toolbar will stay half visible. Actually this is how it’s done in Google Play Games app which I consider as a bug."/> </LinearLayout> <View android:layout_width="wrap_content" android:layout_height="30dp" /> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:text="hai" android:layout_width="160dp" android:layout_height="match_parent" /> <Button android:text="hello" android:layout_width="160dp" android:layout_height="match_parent" /> </LinearLayout>
</android.support.v4.widget.NestedScrollView>
activity_main.XML
<android.support.design.widget.AppBarLayout android:layout_height="wrap_content" android:layout_width="match_parent" android:theme="@style/AppTheme.AppBarOverlay"> <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" app:popupTheme="@style/AppTheme.PopupOverlay" /> </android.support.design.widget.AppBarLayout> <include layout="@layout/content_main" />
-
chanti about 8 yearsI added this line in tool bar but still not getting output app:layout_scrollFlags="scroll|enterAlways"@Aritra Roy
-
chanti about 8 yearswhen i scroll my content my tool bar name visible in status bar
-
chanti about 8 yearsi post my output image ,i use your code in my xml but my output like above@Dhawal Sodha Parmar
-
Dhaval Parmar about 8 years@chanti: remove android:fitsSystemWindows="true" from CoordinatorLayout in activity_main.XML
-
chanti about 8 yearsnow its working after removing i am facing another problem,my status bar color display in white color@Dhawal Sodha Parmar
-
Dhaval Parmar about 8 yearsuse <item name="android:statusBarColor">@color/colorPrimary</item> in style.xml of v21