How to ignore/remove leading zeros?
16,848
Solution 1
To get the actual digits (I assume that each digit is stored as a byte in an array of 20 bytes, lowest digit at highest index), you do something like this:
int i;
int size = sizeof(thearray) / sizeof(thearray[0]);
/* find first non-0 byte, starting at the highest "digit" */
for (i = 0; i < size - 1; ++i)
if (thearray[i] != 0)
break;
/* output every byte as character */
for (; i < size; i++)
printf("%c", thearray[i] + '0'); /* 0 --> '0', 1 --> '1', etc. */
printf("\n");
Solution 2
You can do this by below code:-
int flag=1;
for(i=0;i<20;i++)
{
if(flag==1&&array[i]!=0)
flag=0;
if(flag!=1)
{
printf("%d",array[i]);
}
}
This will remove all leading zeros.
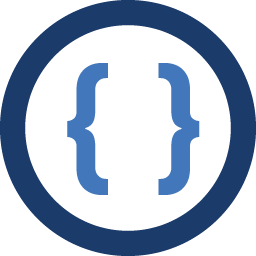
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am writing a program to add two large numbers in
C
. My integer array result holds the sum of the two numbers (which were also stored in arrays).For example, if the result array is
[0,0,3,2]
(actual array size is 20)If 32 is my actual result, how can I display the contents of the result array without the leading zeros ?
#include <stdio.h> #include <string.h> #include <stdlib.h> #define BASE 10 void align(int A[],int n); void add(int A[],int B[], int C[]); void Invert(int* a, int n); int main(int argc, char** argv){ char input1[20]; char input2[20]; int size = 20; int a; int b; int num1[20]; int num2[20]; int result[20]; int length1 = strlen(argv[1]); int length2 = strlen(argv[2]); int i = 0; for (i=0;i<length1;i++){ input1[i] = argv[1][i]; } for (i=0;i<length2;i++){ input2[i] = argv[2][i]; } a=atoi(input1); b=atoi(input2); align(num1,a); align(num2,b); add(num1,num2,result); Invert(result,size); for (i=0;i<20;i++){ printf("%d",result[i]); } return 0; } void align (int A[], int n){ int i = 0; while (n) { A[i++] = n % BASE; n /= BASE; } while (i < 20) A[i++] = 0; } void add (int A[], int B[], int C[]) { int i, carry, sum; carry = 0; for (i=0; i<20; i++) { sum = A[i] + B[i] + carry; if (sum >= BASE) { carry = 1; sum -= BASE; } else carry = 0; C[i] = sum; } if (carry) printf ("overflow in addition!\n"); } void Invert(int* a, int n) { int i; int b; for(i=0; i<n/2; i++){ b = a[i]; a[i] = a[n-i-1]; a[n-i-1] = b; } }
`
-
simonc almost 10 years+1. Note that terminating the first loop at
size-1
would allow you to avoid the laterif else
. Also, might be worth a note that you can only calculatesize
in the way you show if you have access to the array definition, not just a pointer to the array. -
Rudy Velthuis almost 10 years@simonc: you are right, the lowest digit can always be displayed. I'll change the code accordingly.
-
simonc almost 10 yearsWon't this skip the first non-zero digit?
-
A.s. Bhullar almost 10 yearsIn case of 100 it will print 10
-
A.s. Bhullar almost 10 years@simonc not a first non zero but first zero if zero is not leading, isn't it?
-
Jongware almost 10 yearsActually, for C and decimal digits the disclaimer "assuming ASCII" is obsolete. C mandates that the encoding of
'0'
to'9'
digits is consecutive. -
Rudy Velthuis almost 10 yearsIn C, chars and bytes can both be expressed as number or as character. Adding '0' (i.e. 0x30) to 0 turns the number 0 into the character '0' (ASCII 0x30), adding it to 1 returns the character '1' (ASCII 0x31), etc. The printf format
"%c"
expects a char, not a number. Alternatively, I could have used:printf("%d", thearray[i]);
. -
Admin almost 10 years@RudyVelthuis Thanks !