How to implement a REST API Server?
Solution 1
Hey I also was new to the whole API game not too long ago. I found that deploying an ASP.NET Web API with Visual Studio was a great way to start. The template provided by VS (I'm using 2013) makes it really easy to create your own controllers.
If you look up a couple tutorials on HTTP methods, you can really get the hang of molding your controller(s) to your needs. They map well to the CRUD operations which I'm sure you're looking to perform.
You should also be able to find a library in C++ that will allow you to call each of your controller methods and pass/receive serialized JSON/XML objects. Hope this helped, good luck! :)
Solution 2
Restbed offers asynchronous client/server capabilities via ASIO and C++11. We have lots of examples and documentation will be available shortly for those who don't like reading header files.
#include <memory>
#include <cstdlib>
#include <restbed>
using namespace std;
using namespace restbed;
void post_method_handler( const shared_ptr< Session > session )
{
const auto request = session->get_request( );
int content_length = 0;
request->get_header( "Content-Length", content_length );
session->fetch( content_length, [ ]( const shared_ptr< Session > session, const Bytes & body )
{
fprintf( stdout, "%.*s\n", ( int ) body.size( ), body.data( ) );
session->close( OK, "Hello, World!", { { "Content-Length", "13" } } );
} );
}
int main( const int, const char** )
{
auto resource = make_shared< Resource >( );
resource->set_path( "/resource" );
resource->set_method_handler( "POST", post_method_handler );
auto settings = make_shared< Settings >( );
settings->set_port( 1984 );
settings->set_default_header( "Connection", "close" );
Service service;
service.publish( resource );
service.start( settings );
return EXIT_SUCCESS;
}
The next major feature will allow the ability to dependency inject application layers.
auto settings = make_shared< Settings >( );
Service service;
service.add_application_layer( http_10_instance );
service.add_application_layer( http_11_instance );
service.add_application_layer( http2_instance );
service.add_application_layer( spdy_instance );
service.start( settings );
Solution 3
http://pistache.io/ looks a good and modern to me. The hello world is just 9 lines long.
Solution 4
ngrest is a simple REST framework with epoll, codegeneration, command line tool, extensions and other sugar.
It's easy in use and suitable for beginners; written on C++11 and uses CMake for build.
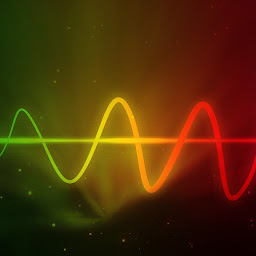
MGItkin
Updated on December 08, 2020Comments
-
MGItkin over 3 years
I am a university student with an intermediate level of C++ programming experience. I would like to implement a simple REST based API for my application as quickly as possible.
I have looked at Casablanca and libWebSockets but the examples posted on their respective sites are a bit over my head. Is there any library that has more beginner oriented tutorials on creating a RESTFUL API Server in C++ ?
Note: I am aware that this question has been asked a few times in C# but the answers are over a year or two old and mostly not aimed at beginners. I hope that a new post will yield some fresh answers!
-
tambre over 7 yearsYou should disclose that you're the creator of the ngrest framework.
-
Jacek Cz about 6 yearsPistache seems in 2018 not supported on Windows?
-
CaTx almost 6 yearsI tried that library but could not access it from another computer. It works locally though. Any pointer to fix this?/
-
CaTx almost 6 yearsI tried installing it but it failed to build.
-
CaTx almost 6 yearsI also tried that library but could not access it from another computer. It works locally though. Any pointer to fix this?
-
Matthew Cornelisse almost 2 yearsdomain has expired