How to implement SearchBar and search display controller in tableview in xib
Solution 1
Here is a sample code
NSMutableArray *filteredResult; // this holds filtered data source
NSMutableArray *tableData; //this holds actual data source
-(void) filterForSearchText:(NSString *) text scope:(NSString *) scope
{
[filteredResult removeAllObjects]; // clearing filter array
NSPredicate *filterPredicate = [NSPredicate predicateWithFormat:@"SELF.restaurantName contains[c] %@",text]; // Creating filter condition
filteredResult = [NSMutableArray arrayWithArray:[tableData filteredArrayUsingPredicate:filterPredicate]]; // filtering result
}
Delegate Methods
-(BOOL) searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchString:(NSString *)searchString
{
[self filterForSearchText:searchString scope:[[[[self searchDisplayController] searchBar] scopeButtonTitles] objectAtIndex:[[[self searchDisplayController] searchBar] selectedScopeButtonIndex] ]];
return YES;
}
-(BOOL) searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchScope:(NSInteger)searchOption
{
[self filterForSearchText:self.searchDisplayController.searchBar.text scope:
[[self.searchDisplayController.searchBar scopeButtonTitles] objectAtIndex:searchOption]];
return YES;
}
In NSPredicate condition "@"SELF.restaurantName contains[c] %@",text " restaurantName is a property name which needs to filtered against. If you have only NSString in your datasource array, you can use like @"SELF contains[c] %@",text
Once the filter is done, then you need to implement your tableview delegate accordingly. Something like this
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
if(tableView == [[self searchDisplayController] searchResultsTableView])
{
return [filteredResult count];
}
else
{
return [tableData count];
}
}
compare the tableview whether it is filtered tableview or original tableview and set the delegate and datasource for tableview accordingly.Please note, searchDisplayController is available property for UIViewcontroller and we can just use it to display filtered result.
For above code to work, you need to use "Search Bar and Search Display" object if you are using it in a XIB or storyboard
Solution 2
Refer the following sample codes,
http://www.appcoda.com/how-to-add-search-bar-uitableview/
These examples may give you a better idea
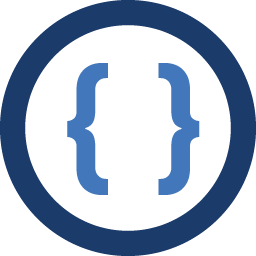
Admin
Updated on August 10, 2020Comments
-
Admin over 3 years
I have mutable array with dictionaries.I`am displaying that array in table view.
Now i want to implement search and display controller to table view. How?
Any suggestions or code..
Here my array i`am displaying "name" key in uitableview as alphabetically order.
[ { "name": "Fish", "description": "sdhshs", "colorCode": null, }, { "name": "fry", "description": "sdhshs", "colorCode": null, }, { "name": "curry", "description": "sdhshs", "colorCode": null, } ],
-
Admin over 10 yearsI have mutable array which has dictionaries. can u tell me how to display
-
slysid over 10 yearsCan you give a sample of your Array of Dictionary and on what condition you are trying for filtered out in dictionary? For example, in array of say first names, I can filter out using alphabets starting with A or B or C, something like a condition on which you want to filter.
-
Admin over 10 yearsPlz,check my edited question..i updated
-
slysid over 10 yearsgithub.com/slysid/iOS/tree/master/DictSearch Look for sample code for the data yo have provided. The search will filter for name. i.e f will filter out fish and fry in your filter result.
-
Admin over 10 yearssearch is working properly...but cells is not displaying properly.it`s overlapping cell on cell.
-
slysid over 10 yearsIs overlapping occurs in sample project I have given? It looks fine for me. Moreover, the code is to explain and how to use search using array of dicts. Any overlap of cells, may be due frame size not set properly.
-
Admin over 10 yearsI implemented your logic in my project.When i start search in searchBar all cells of search result are overlapping each other.
-
slysid over 10 yearsHave you implemented any custom tableview cell font or text size in it? Try to implement - (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath and see if the tableview is search result, then return a higher row height value.