How to initialize a a struct of structs inside a header file in C?
Solution 1
You shouldn't instantiate any structures in header files. If you do a different instance will be created in each C file you include the header in which is usually not the desired effect.
In a C file to do this you would have to do the following.
void foo(){
struct A parent;
struct B child_b;
struct C child_c;
child_b.temp3 = 3;
child_b.temp4 = 4;
child_b.temp5 = 5;
child_c.temp6 = 6;
child_c.temp7 = 7;
child_c.temp8 = 8;
parent.temp1 = child_b;
parent.temp2 = child_c;
}
I would strong consider making helper functions similar to this
void initB(struct B* s, int x, int y, int z){
s->temp3 = x;
s->temp4 = y;
s->temp5 = z;
}
If you would like the keep the array initialization syntax then consider using a union.
Solution 2
Declare struct B and C before A, i.e. :
struct B { int temp3; int temp4; int temp5; };
struct C { int temp6; int temp7; int temp8; };
struct A { struct B temp1; struct C temp2; };
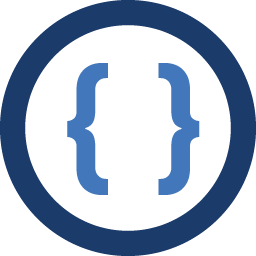
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am trying to port some old code over from a 20 year old DOS system to a GNU Linux system. In several of their header files, (which are included all over the place), they have structs of structs that they declare and initialize. I am getting warnings when I compile with the way the legacy code was written. Any tips on how I can get this to work with staying inside the same header file?
The following is a simplified example I made of what they are doing.
struct A { struct B temp1; struct C temp2; }; struct B { int temp3; int temp4; int temp5; }; struct C { int temp6; int temp7; int temp8; }; //These are the variables in how they are related to the initialization below //struct A test_one = {{temp3,temp4,temp5},{temp6,temp7,temp8}}; struct A test_one = {{1,2,3},{4,5,6}};