How to initialize firebase crashlytics in Flutter?
Yes, your configuration of the crashlytics is ok.
If you are using Firebase Auth, you can add the following code in order to have the ability to track crashes specific to a user:
FirebaseAuth.instance.onAuthStateChanged.listen((firebaseUser) {
if (firebaseUser != null && firebaseUser?.email != null) {
Crashlytics.instance.setUserEmail(firebaseUser.email);
}
if (firebaseUser != null && firebaseUser?.uid != null) {
Crashlytics.instance.setUserIdentifier(firebaseUser.uid);
}
if (firebaseUser != null && firebaseUser?.displayName != null) {
Crashlytics.instance.setUserName(firebaseUser.displayName);
}
});
Also, don't forget to track specific exceptions in catch of the try-catch block like this:
try {
//some code here...
} catch (e, s) {
Crashlytics.instance.recordError(e, s, context: "an error occured: uid:$uid");
}
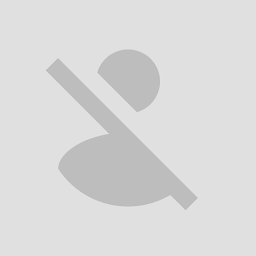
TB13
Updated on December 24, 2022Comments
-
TB13 over 1 year
I have already implemented firebase crashlytics to my Flutter project through dependency in pubspec.yaml and also in Gradle files and able to see the crashlytics dashboard in the firebase console.
Now my question is how can I initialize crashlytics in main.dart file and how to write log and catch error or crash for a particular page(say Home page).
I have tried from this link: https://pub.dev/packages/firebase_crashlytics/example
main.dart
final _kShouldTestAsyncErrorOnInit = false; // Toggle this for testing Crashlytics in your app locally. final _kTestingCrashlytics = true; main() { WidgetsFlutterBinding.ensureInitialized(); runZonedGuarded(() { runApp(MyApp()); }, (error, stackTrace) { print('runZonedGuarded: Caught error in my root zone.'); FirebaseCrashlytics.instance.recordError(error, stackTrace); }); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: "My App", debugShowCheckedModeBanner: false, home: MainPage(), theme: ThemeData( accentColor: Colors.blue ), ); } } class MainPage extends StatefulWidget { @override _MainPageState createState() => _MainPageState(); } class _MainPageState extends State<MainPage> { Future<void> _initializeFlutterFireFuture; Future<void> _testAsyncErrorOnInit() async { Future<void>.delayed(const Duration(seconds: 2), () { final List<int> list = <int>[]; print(list[100]); }); } // Define an async function to initialize FlutterFire Future<void> _initializeFlutterFire() async { // Wait for Firebase to initialize await Firebase.initializeApp(); if (_kTestingCrashlytics) { // Force enable crashlytics collection enabled if we're testing it. await FirebaseCrashlytics.instance.setCrashlyticsCollectionEnabled(true); } else { // Else only enable it in non-debug builds. // You could additionally extend this to allow users to opt-in. await FirebaseCrashlytics.instance .setCrashlyticsCollectionEnabled(!kDebugMode); } // Pass all uncaught errors to Crashlytics. Function originalOnError = FlutterError.onError; FlutterError.onError = (FlutterErrorDetails errorDetails) async { await FirebaseCrashlytics.instance.recordFlutterError(errorDetails); // Forward to original handler. originalOnError(errorDetails); }; if (_kShouldTestAsyncErrorOnInit) { await _testAsyncErrorOnInit(); } } @override void initState() { super.initState(); _initializeFlutterFireFuture = _initializeFlutterFire(); Firebase.initializeApp().whenComplete(() { print("completed"); setState(() {}); }); checkLoginStatus(); } }
Is it correct or any otherway to initialize crashlytics in flutter? If i have to check whether there is any crash in HomePage, then how can i get that crash from home page and will show it in firbase crashlytics?