How to initialize std::unique_ptr in constructor?
44,216
Solution 1
You need to initialize it through the member-initializer list:
A::A(std::string filename) :
file(new std::ifstream(filename));
{ }
Your example was an attempt to call operator ()
on a unique_ptr
which is not possible.
Update: BTW, C++14 has std::make_unique
:
A::A(std::string filename) :
file(std::make_unique<std::ifstream>(filename));
{ }
Solution 2
You can do it like this:
A:A(std::string filename)
: file(new std::ifstream(filename.c_str())
{
}
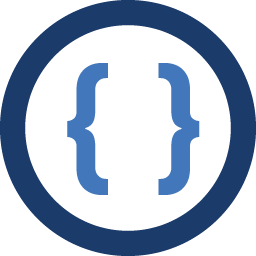
Author by
Admin
Updated on May 25, 2020Comments
-
Admin almost 4 years
A.hpp:
class A { private: std::unique_ptr<std::ifstream> file; public: A(std::string filename); };
A.cpp:
A::A(std::string filename) { this->file(new std::ifstream(filename.c_str())); }
The error that I get is thrown:
A.cpp:7:43: error: no match for call to ‘(std::unique_ptr<std::basic_ifstream<char> >) (std::ifstream*)’
Does anyone have any insight as to why this is occurring? I've tried many different ways to get this to work but to no avail.
-
gigaplex over 10 yearsAlternatively call the
reset()
function onfile
to assign theunique_ptr
if you need to do some checking in the constructor beforehand. -
JohnJohn over 9 yearsYou don't necessarily have to use member-initializer list. It is more preferable though.
-
csguth over 7 years@JohnJohn, if you're using Pimpl, you should use member-initializer list, since your std::unique_ptr is const.
-
Dirk Herrmann over 3 yearsRegarding the use of
file(new ...)
vs.file(std::make_unique...)
: Is there any reason to prefer one over the other?