How to insert in 2 or more tables using Transaction
1,230
You can use entityManager
for create transaction, like this :
export class ExampleTransaction {
constructor(
@InjectEntityManager()
private readonly entityManager: EntityManager,
) { }
public async transactionExampleMethod(): Promise<void> {
await this.entityManager.transaction(async (transactionalManager: EntityManager) => {
//## SHIPMENT ENTITY ##
const shipmentToInsert = Transformador.prepareShipment(ShipConfirmDetails, ShipConfirmSummary);
const shipmentInserted = await transactionalManager.save(shipmentToInsert);
//## ORDER ENTITY ##
const ordersToInsert = Transformador.prepareOrder(ShipConfirmDetails, shipmentInserted.shipment_id)
const orderedInserted = await transactionalManager.save(ordersToInsert)
//## LINE ENTITY ##
const linesToInsert = Transformador.prepareLines(ShipConfirmDetails, orderedInserted)
const linesInserted = await transactionalManager.save(linesToInsert)
}
}
}
Related videos on Youtube
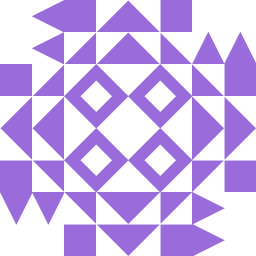
Author by
Programmer89
Updated on January 01, 2023Comments
-
Programmer89 20 minutes
I need to do some actions that if all pass it commits or all fails.
I know that transactions could do this but I'm having trouble with multiple table inserts.
The documentation of nestJs says I only need to import the Connect method and create a specific structure but, it does not cover when it's more than 1 table.
NestJs Docu Code:
async createMany(users: User[]) { const queryRunner = this.connection.createQueryRunner(); await queryRunner.connect(); await queryRunner.startTransaction(); try { await queryRunner.manager.save(users[0]); await queryRunner.manager.save(users[1]); await queryRunner.commitTransaction(); } catch (err) { // since we have errors lets rollback the changes we made await queryRunner.rollbackTransaction(); } finally { // you need to release a queryRunner which was manually instantiated await queryRunner.release(); } }
As you can see all that refers to the User Entity, where does he specify that?
My code:
const queryR = this.connection.createQueryRunner(); await queryR.connect(); await queryR.startTransaction(); try { //## SHIPMENT ENTITY ## const shipmentToInsert = Transformador.prepareShipment(ShipConfirmDetails, ShipConfirmSummary) //const shipmentInserted = await this.shipmentRepositoryService.insert(shipmentToInsert)//normal insert of entity const shipmentInserted = await queryR.manager.save(shipmentToInsert) //## ORDER ENTITY ## const ordersToInsert = Transformador.prepareOrder(ShipConfirmDetails, shipmentInserted.shipment_id) const orderedInserted = await queryR.manager.save(ordersToInsert) //## LINE ENTITY ## const linesToInsert = Transformador.prepareLines(ShipConfirmDetails, orderedInserted) const linesInserted = await queryR.manager.save(linesToInsert) } catch (e) { console.log(">>Error, se procede a hacer Rollback>>"); await queryR.rollbackTransaction(); } finally { console.log(">>Se libera Transaction>>"); await queryR.release(); }