How To integrate Aadhaar Card Authentication Api for Aadhaar number Verification in PHP?
25,090
Solution 1
// Below is the running code to integrate Aadhaar Card Authentication Api for Aadhaar number Verification in PHP?
$path=$_SERVER['DOCUMENT_ROOT'];
$certpath=$path."/your .pfx file";
$publickeypath=$path."/your .cer file";
$certpassword="your cert password";
require_once('xmlsecurity.php'); // for creating this file use link : https://github.com/robrichards/xmlseclibs
$trn_id = "AuthDemoClient:public:". date('YmdHisU');
if (!$cert_store = file_get_contents($certpath)) {
echo "Error: Unable to read the cert file\n";
exit;
}
if (openssl_pkcs12_read($cert_store, $cert_info, $certpassword)) {
//print_r($cert_info["cert"]);
//print_r($cert_info["pkey"]);
} else {
echo "Error: Unable to read the cert store.\n";
exit;
}
define("UIDAI_PUBLIC_CERTIFICATE" , $publickeypath);
define("AUA_PRIVATE_CERTIFICATE" , $cert_info["pkey"]);
date_default_timezone_set("Asia/Calcutta");
$date2= gmdate("Y-m-d\TH:i:s");
$date1 = date('Y-m-d\TH:i:s', time());
$ts='"'.$date1.'"';//date('Y-m-d\TH:i:s');
$pid_1='<Pid ts='.$ts.' ver="1.0"><Pv otp="'.$otp.'"/></Pid>';
$randkey = generateRandomString();
$SESSION_ID = $randkey;
$skey1=encryptMcrypt($SESSION_ID);
$skey=base64_encode($skey1);
// generate ci code start
$ci=getExpiryDate(UIDAI_PUBLIC_CERTIFICATE);
// generate pid block code start
$pid=encryptPID($pid_1,$randkey);
//hmac creation code start
$hash=hash("SHA256",$pid_1,true);
$hmac=encryptPID($hash,$randkey);
$load_xml="<?xml version=\"1.0\" encoding=\"UTF-8\" standalone=\"yes\"?><Auth xmlns=\"http://www.uidai.gov.in/authentication/uid-auth-request/1.0\" sa=\"public\" lk=\"your license key\" txn=\"$trn_id\" ver=\"1.6\" tid=\"public\" ac=\"your code from aadhaar\" uid=\"$aadhaarno\"><Uses pi=\"n\" pa=\"n\" pfa=\"n\" bio=\"n\" bt=\"\" pin=\"n\" otp=\"y\"/><Meta udc=\"UDC:001\" fdc=\"NC\" idc=\"NA\" pip=\"NA\" lot=\"P\" lov=\"$pincode\"/><Skey ci=\"$ci\">$skey</Skey><Data type=\"X\">$pid</Data><Hmac>$hmac</Hmac></Auth>";
$dom = new DOMDocument();
$dom->loadXML($load_xml); // the XML you specified above.
$objDSig = new XMLSecurityDSig();
$objDSig->setCanonicalMethod(XMLSecurityDSig::C14N_COMMENTS);
$objDSig->addReference($dom, XMLSecurityDSig::SHA1, array('http://www.w3.org/2000/09/xmldsig#enveloped-signature'),array('force_uri'
=>'true'));
$objKey = new XMLSecurityKey(XMLSecurityKey::RSA_SHA1, array('type'=>'private'));
$objKey->loadKey($cert_info["pkey"], False);
$objKey->passphrase = 'your certificate password';
$objDSig->sign($objKey, $dom->documentElement);
$objDSig->add509Cert($cert_info["cert"]);
$objDSig->appendSignature($dom->documentElement);
$xml_string = $dom->saveXML();
$xml_string1 = urlencode($xml_string);
$curl = curl_init();
$url=""; //aadhar service url
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($curl, CURLOPT_POSTFIELDS,"eXml=A28".$xml_string1);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
/* complete within 20 seconds */
curl_setopt($curl, CURLOPT_TIMEOUT, 20);
$result = curl_exec($curl);
curl_close($curl);
$xml = @simplexml_load_string($result);
$return_status=$xml['ret'];
if($return_status=="y"){
$res=1;
}
if($return_status!="y"){
$res=0;
}
}else
{
$res='Aadhaarno not exist';
}
return array('Message'=>$res);
}
function encryptMcrypt($data) {
$fp=fopen(UIDAI_PUBLIC_CERTIFICATE,"r");
$pub_key_string=fread($fp,8192);
openssl_public_encrypt($data, $encrypted_data, $pub_key_string, OPENSSL_PKCS1_PADDING);
return $encrypted_data;
}
function generateRandomString($length = 32) {
$characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
$charactersLength = strlen($characters);
$randomString = '';
for ($i = 0; $i < $length; $i++) {
$randomString .= $characters[rand(0, $charactersLength - 1)];
}
return $randomString;
}
function encryptPID($data,$skey) {
$result=openssl_encrypt ( $data , 'AES-256-ECB' , $skey );
return ($result);
}
function getExpiryDate($_CERTIFICATE){
$_CERT_DATA = openssl_x509_parse(file_get_contents($_CERTIFICATE));
return date('Ymd', $_CERT_DATA['validTo_time_t']);
}
Solution 2
To generate the XML, you could use this library to generate it quite easily:
https://github.com/iwyg/xmlbuilder
For the encryption; I could be wrong but it looks like (from this page) you can generate the encoded results in java one time and just paste in the results to your PHP variables.
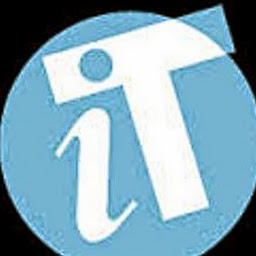
Comments
-
ITit superpower about 3 years
I want to integrate the Aadhaar Card Authentication API for Aadhaar number Verification in PHP. I tried this and wrote code for API access.
How can I generate the XML encryption field data below in PHP? This sample code is written in Java.
<Auth uid="" tid="" ac="" sa="" ver="" txn="" lk=""> <Uses pi="" pa="" pfa="" bio="" bt="" pin="" otp=""/> <Tkn type="" value=""/> <Meta udc="" fdc="" idc="" pip="" lot=”G|P” lov=""/> <Skey ci="" ki="">encrypted and encoded session key</Skey> <Data type=”X|P”>encrypted PID block</Data> <Hmac>SHA-256 Hash of Pid block, encrypted and then encoded</Hmac> <Signature>Digital signature of AUA</Signature> </Auth>
-
Ravi Yadav about 8 yearsI want to integrate just aadhar status and download adhar into my android app...so can you guide how to send parameters to apis and which api urls to be used?
-
Jagdeep chauhan about 7 yearsAbove code is to integrate Aadhaar Card Authentication Api for Aadhaar number Verification in PHP after getting otp from GETOTP webservice.
-
Lokesh Pandey about 7 yearsHi can you please tell me how could i get the values of encrypted and encoded session key in Skey, encrypted PID block in data, SHA-256 Hash of Pid block, encrypted and then encoded in Hmac and Digital signature in Signature ? I have gone through the documentation but no idea how could i got these values
-
Jagdeep chauhan almost 7 years@lokesh : Answer of all your questions is already shared in above code. Above code includes, 1. encrypted and encoded session key in Skey. 2. encrypted PID block in data, SHA-256 Hash of Pid block 3. encrypted and then encoded in Hmac. 4. Creation of signed xml using digital signatures.
-
Lokesh Pandey almost 7 yearsThank you for writing, what is xmlsecurity.php in your code ?
-
Jagdeep chauhan almost 7 years@lokesh : xml security.php is a PHP library for XML Security which is used to create a signed xml using certificate private key and password (github.com/robrichards/xmlseclibs). This signed xml will be the request xml for aadhaar service URL.
-
s4suryapal almost 7 years@ Jagdeep chauhan, hi , is it possible to get aadhar card using this Auth API ?
-
Jagdeep chauhan almost 7 years@s4suryapal : You can not get aadhaar, you can validate aadhaar using this API, You have to pass aadhaar and OTP in request.
-
saravana over 6 yearsHi, where can I get .pfx and .cer files?
-
Jagdeep chauhan over 6 years@saravana : From Aadhar Based Digital Signature Certificate Providers like e-mudra.
-
swapnil kambe almost 6 yearsHow could I get pfx file