How to iterate over a TreeMap?
325,171
Solution 1
Assuming type TreeMap<String,Integer> :
for(Map.Entry<String,Integer> entry : treeMap.entrySet()) {
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println(key + " => " + value);
}
(key and Value types can be any class of course)
Solution 2
//create TreeMap instance
TreeMap treeMap = new TreeMap();
//add key value pairs to TreeMap
treeMap.put("1","One");
treeMap.put("2","Two");
treeMap.put("3","Three");
/*
get Collection of values contained in TreeMap using
Collection values()
*/
Collection c = treeMap.values();
//obtain an Iterator for Collection
Iterator itr = c.iterator();
//iterate through TreeMap values iterator
while(itr.hasNext())
System.out.println(itr.next());
or:
for (Map.Entry<K,V> entry : treeMap.entrySet()) {
V value = entry.getValue();
K key = entry.getKey();
}
or:
// Use iterator to display the keys and associated values
System.out.println("Map Values Before: ");
Set keys = map.keySet();
for (Iterator i = keys.iterator(); i.hasNext();) {
Integer key = (Integer) i.next();
String value = (String) map.get(key);
System.out.println(key + " = " + value);
}
Solution 3
Just to point out the generic way to iterate over any map:
private <K, V> void iterateOverMap(Map<K, V> map) {
for (Map.Entry<K, V> entry : map.entrySet()) {
System.out.println("key ->" + entry.getKey() + ", value->" + entry.getValue());
}
}
Solution 4
Using Google Collections, assuming K is your key type:
Maps.filterKeys(treeMap, new Predicate<K>() {
@Override
public boolean apply(K key) {
return false; //return true here if you need the entry to be in your new map
}});
You can use filterEntries
instead if you need the value as well.
Related videos on Youtube
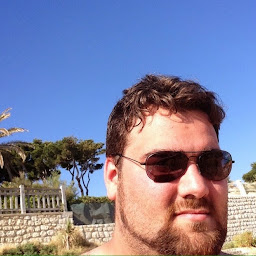
Comments
-
gregory boero.teyssier about 4 years
Possible Duplicate:
How do I iterate over each Entry in a Map?I want to iterate over a
TreeMap
, and for all keys which have a particular value, I want them to be added to a newTreeMap
. How can I do this?-
Stephen C over 14 years@Click: "... for all 'key's which have a particular value ...". Do you mean for all keys in a given Set, or for all keys that satisfy a given predicate?
-
-
Esko over 14 yearsFYI, EntrySet is the preferred way to iterate through any Map since EntrySets are by specification reflective and always represent the state of data in the Map even if the Map underneath would change.
-
SamDJava over 9 yearsAdding to Zed's answer , using entrySet will give the user the power to delete a particular entry while iterating.
-
intcreator over 8 yearsGiving the name
treeMap
to yourTreeMap
threw me off at first. -
phil294 over 7 yearswill this give out the values ordered?
-
zyamys over 7 yearsThis doesn't do any sorting.
-
André Luiz Reis about 6 yearsNo. TreeMap order by Key value.
-
ericcurtin about 4 yearsThere must be a more performant way that do another treemap lookup here: "(String) map.get(key)". You already know the element at this stage, just curious.