How to Kill A Session or Session ID (ASP.NET/C#)
Solution 1
The Abandon
method should work (MSDN):
Session.Abandon();
If you want to remove a specific item from the session use (MSDN):
Session.Remove("YourItem");
EDIT: If you just want to clear a value you can do:
Session["YourItem"] = null;
If you want to clear all keys do:
Session.Clear();
If none of these are working for you then something fishy is going on. I would check to see where you are assigning the value and verify that it is not getting reassigned after you clear the value.
Simple check do:
Session["YourKey"] = "Test"; // creates the key
Session.Remove("YourKey"); // removes the key
bool gone = (Session["YourKey"] == null); // tests that the remove worked
Solution 2
It is also a good idea to instruct the client browser to clear session id cookie value.
Session.Clear();
Session.Abandon();
Response.Cookies["ASP.NET_SessionId"].Value = string.Empty;
Response.Cookies["ASP.NET_SessionId"].Expires = DateTime.Now.AddMonths(-10);
Solution 3
This marks the session as Abandoned, but the session won't actually be Abandoned at that moment, the request has to complete first.
Solution 4
From what I tested:
Session.Abandon(); // Does nothing
Session.Clear(); // Removes the data contained in the session
Example:
001: Session["test"] = "test";
002: Session.Abandon();
003: Print(Session["test"]); // Outputs: "test"
Session.Abandon does only set a boolean flag in the session-object to true. The calling web-server may react to that or not, but there is NO immediate action caused by ASP. (I checked that myself with the .net-Reflector)
In fact, you can continue working with the old session, by hitting the browser's back button once, and continue browsing across the website normally.
So, to conclude this: Use Session.Clear() and save frustration.
Remark: I've tested this behaviour on the ASP.net development server. The actual IIS may behave differently.
Solution 5
Session.Abandon();
did not work for me either.
The way I had to write it to get it to work was like this. Might work for you too.
HttpContext.Current.Session.Abandon();
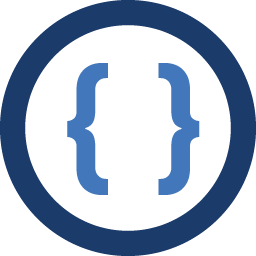
Admin
Updated on February 05, 2021Comments
-
Admin over 3 years
How can I destroy a session (Session["Name"]) when the user clicks the logout button?
I'm looking through the ASP.NET API Reference on MSDN and it doesn't seem to have much information. It seems rather limited. But I cannot find any other pages for ASP.NET Classes etc.
I have tried:
Session.Abandon();
andSession.Contents.Remove("Name");
neither of them work. ( I found these in a forum from a Google search)