How to let OnClick in ListView's Adapter call Activity's function
Solution 1
Inside onClick()
Do something like this:
((ParentClass) context).functionToRun();
Solution 2
Just for clarity to expand on provided answers
In a BaseAdapter you can get the parent class by calling this.getActivity();
If you then typecast this to the actual activity class you can then call a function as per @AdilSoomro answer below so you actually end up with something like this
public class MyAdapter extends BaseAdapter<Long> {
public MyAdapter(Activity activity,
TreeStateManager<Long> treeStateManager, int numberOfLevels) {
super(activity, treeStateManager, numberOfLevels);
}
@Override
public void handleItemClick(final View view, final Object id) {
((MyActivity) this.activity).someFunction();
}
}
Then just declare someFunction in MyActivity to do what you want
protected void someFunction(){
// Do something here
}
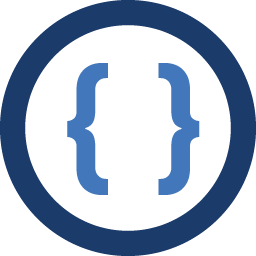
Admin
Updated on October 25, 2020Comments
-
Admin over 3 years
I have an Android application with a ListView in it, the ListView will setup fine but now I want a image in the ListView to be clickable. I do this by using 2 classes, the Activity class (parent) and an ArrayAdapter to fill the list. In the ArrayAdapter I implement a OnClickListener for the image in the list that I want to be clickable.
So far it all works.
But now I want to run a function from the activity class when the onClick, for the image in the list, is run but I do not know how. Below are the 2 classes that I use.
First the Activity class:
public class parent_class extends Activity implements OnClickListener, OnItemClickListener { child_class_list myList; ListView myListView; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); // setup the Homelist data myList = new child_class_list (this, Group_Names, Group_Dates); myListView = (ListView) findViewById(R.id.list); // set the HomeList myListView.setAdapter( myList ); myListView.setOnItemClickListener(this); } void function_to_run() { // I want to run this function from the LiscView Onclick } public void onItemClick(AdapterView<?> arg0, View arg1, int arg2, long arg3) { // do something } }
And the ArrayAdapter from where I want to call a function from the Activity class:
public class child_class_list extends ArrayAdapter<String> { // private private final Context context; private String[] mName; private String[] mDate; public child_class_list (Context context, String[] Name, String[] Date) { super(context, R.layout.l_home, GroupName); this.context = context; this.mName = Name; this.mDate = Date; } @Override public View getView(int position, View convertView, ViewGroup parent) { LayoutInflater inflater = (LayoutInflater) context .getSystemService(Context.LAYOUT_INFLATER_SERVICE); View rowView = inflater.inflate(R.layout.l_home, parent, false); ImageView selectable_image = (ImageView) rowView.findViewById(R.id.l_selectable_image); selectable_image.setOnClickListener(new OnClickListener() { public void onClick(View v) { // I want to run the function_to_run() function from the parant class here } } ); // get the textID's TextView tvName = (TextView) rowView.findViewById(R.id.l_name); TextView tvDate = (TextView) rowView.findViewById(R.id.l_date); // set the text tvName.setText (mName[position]); tvDate.setText (mDate[position]); return rowView; } }
If anyone knows how to run the function in the activity class from the arrayadapter or how to set the image onClickListener in the Activity Class I would greatly apriciate the help.