How to list all folders and their subdirectories/files in iPhone SDK?
Solution 1
Take into account that your app runs in a sandbox and would not be able to get any folder/file outside of that sandbox.
ObjectiveC
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSFileManager *manager = [NSFileManager defaultManager];
NSArray *fileList = [manager contentsOfDirectoryAtPath:documentsDirectory error:nil];
for (NSString *s in fileList){
NSLog(@"%@", s);
}
Swift 4
guard let documentsDirectory = try? FileManager().url(for: .documentDirectory, in: .userDomainMask, appropriateFor: nil, create: true) else { return }
guard let fileEnumerator = FileManager.default.enumerator(at: documentsDirectory, includingPropertiesForKeys: nil, options: FileManager.DirectoryEnumerationOptions()) else { return }
while let file = fileEnumerator.nextObject() {
print(file)
}
Solution 2
Here's the slowest(?) approach:
NSFileManager * fileManager = [NSFileManager new];
NSArray * subpaths = [fileManager subpathsAtPath:path];
but that should at least point you to a more specialized implementation for your needs.
Slightly lower level abstractions which allow you to enumerate on demand include NSDirectoryEnumerator
and CFURLEnumerator
. Depending on the depth of the directory, these have the potential to save much unnecessary interactions with the filesystem, compared to -[NSFileManager subpathsAtPath:]
.
Solution 3
You can use NSDirectoryEnumerator
via NSFileManager.enumeratorAtPath
From the docs:
NSString *docsDir = [NSHomeDirectory() stringByAppendingPathComponent: @"Documents"];
NSFileManager *localFileManager=[[NSFileManager alloc] init];
NSDirectoryEnumerator *dirEnum =
[localFileManager enumeratorAtPath:docsDir];
NSString *file;
while ((file = [dirEnum nextObject])) {
if ([[file pathExtension] isEqualToString: @"doc"]) {
// process the document
[self scanDocument: [docsDir stringByAppendingPathComponent:file]];
}
}
Solution 4
swift 3
let fileManager:FileManager = FileManager()
let files = fileManager.enumerator(atPath: NSHomeDirectory())
while let file = files?.nextObject() {
print("Files::",file)
}
Solution 5
I'm an author of FileExplorer control which is a file browser for iOS and fulfills most of your requirements. Note that it allows you to browse only those files and directories that are placed inside your sandbox.
Here are some of the features of my control:
- Possibility to choose files or/and directories if there is a need for that
- Possiblity to remove files or/and directories if there is a need for that
- Built-in search functionality
- View Audio, Video, Image and PDF files.
- Possibility to add support for any file type.
You can find my control here.
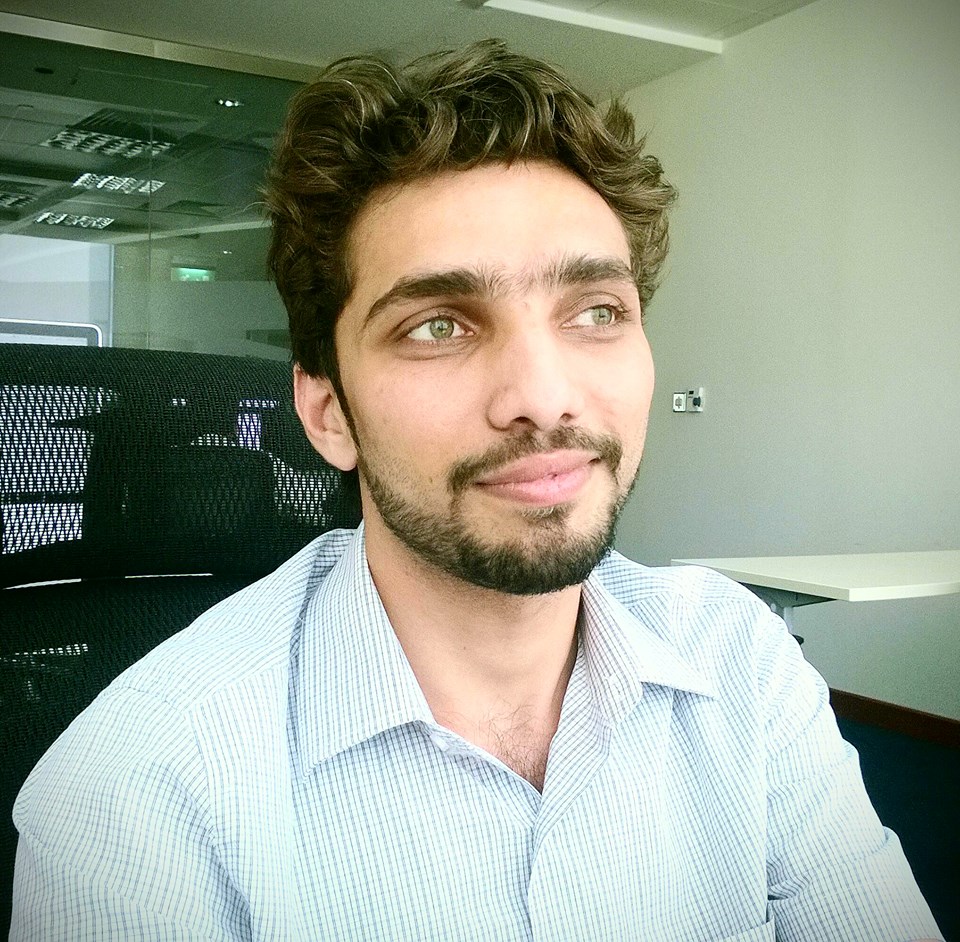
Comments
-
Muhammad Saqib 7 months
I want the user to select any file present in her/his iPhone so that it’s used as an e-mail attachment. For this purpose, I want to show the list of all files and folders present in iPhone. But how would I get the list of those files? Is there any way to get that list?
-
Muhammad Saqib about 11 yearsCan u please mention the value of the variable "path" in the second line.
-
justin about 11 years@Muhammed Well, you don't have access to any file anywhere on the filesystem because it is sandboxed. You do have access to your app's local data -- simpleBob's
documentsDirectory
demonstrates how you would get that path. -
Muhammad Saqib about 11 yearsThank you for your response one small confusion. what if my files is present in the photos folder of iPhone. Do i access it ? i mean to say that can i list down all the files present in the photos folder.
-
justin about 11 years@Muhammed Saqib you're welcome. for those shared images, you can use AssetsLibrary.framework.