How to load a json data file into a variable in robot framework?
Solution 1
One way would be to use the Get File keyword from the OperatingSystem library, and then use the built-in Evaluate keyword to convert it to a python object.
For example, consider a file named example.json with the following contents:
{
"firstname": "Inigo",
"lastname": "Montoya"
}
You can log the name with something like this:
*** Settings ***
| Library | OperatingSystem
*** Test Cases ***
| Example of how to load JSON
| | # read the raw data
| | ${json}= | Get file | example.json
| |
| | # convert the data to a python object
| | ${object}= | Evaluate | json.loads('''${json}''') | json
| |
| | # log the data
| | log | Hello, my name is ${object["firstname"]} ${object["lastname"]} | WARN
Of course, you could also write your own library in python to create a keyword that does the same thing.
Solution 2
There is a library available for this: HttpLibrary.HTTP
${json}= | Get file | example.json
${port} | HttpLibrary.HTTP.Get Json Value | ${json} | /port
log | ${port}
API Document is available here: http://peritus.github.io/robotframework-httplibrary/HttpLibrary.html
Solution 3
A common use is passing the json data to another library like Http Library Requests. You could do:
*** Settings ***
Library OperatingSystem
Library RequestsLibrary
*** Test Cases ****
Create User
#...
${file_data}=
... Get Binary File ${RESOURCES}${/}normal_user.json
Post Request example_session /user data=${file_data}
#...
No direct python involved and no intermediary json object.
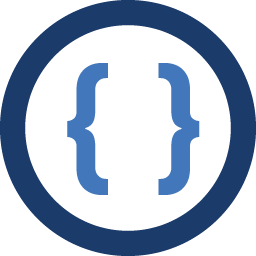
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I Am trying to load a json data file into a variable directly in Robot Framework. Can anyone please elaborate with an e.g. giving the exact syntax as to how to do it? Thanks in advance :)
-
Raceyman almost 10 yearsYou could, also, skip the Get File altogether if desired:
${object} | Evaluate | json.load(open("path_to_json_file", "r")) | json
-
Russell Smith almost 10 years@Raceyman: you are correct. I don't recall why that didn't occur to me earlier. Thanks!