How to load up CSS files using Javascript?
Solution 1
If you use jquery:
$('head').append('<link rel="stylesheet" type="text/css" href="style.css">');
Solution 2
I guess something like this script would do:
<script type="text/javascript" src="/js/styles.js"></script>
This JS file contains the following statement:
if (!document.getElementById) document.write('<link rel="stylesheet" type="text/css" href="/css/versions4.css">');
The address of the javascript and css would need to be absolute if they are to refer to your site.
Many CSS import techniques are discussed in this "Say no to CSS hacks with branching techniques" article.
But the "Using JavaScript to dynamically add Portlet CSS stylesheets" article mentions also the CreateStyleSheet possibility (proprietary method for IE):
<script type="text/javascript">
//<![CDATA[
if(document.createStyleSheet) {
document.createStyleSheet('http://server/stylesheet.css');
}
else {
var styles = "@import url(' http://server/stylesheet.css ');";
var newSS=document.createElement('link');
newSS.rel='stylesheet';
newSS.href='data:text/css,'+escape(styles);
document.getElementsByTagName("head")[0].appendChild(newSS);
}
//]]>
Solution 3
Element.insertAdjacentHTML has very good browser support, and can add a stylesheet in one line.
document.getElementsByTagName("head")[0].insertAdjacentHTML(
"beforeend",
"<link rel=\"stylesheet\" href=\"path/to/style.css\" />");
Solution 4
If you want to know (or wait) until the style itself has loaded this works:
// this will work in IE 10, 11 and Safari/Chrome/Firefox/Edge
// add ES6 poly-fill for the Promise, if needed (or rewrite to use a callback)
let fetchStyle = function(url) {
return new Promise((resolve, reject) => {
let link = document.createElement('link');
link.type = 'text/css';
link.rel = 'stylesheet';
link.onload = function() { resolve(); console.log('style has loaded'); };
link.href = url;
let headScript = document.querySelector('script');
headScript.parentNode.insertBefore(link, headScript);
});
};
Solution 5
In a modern browser you can use promise
like this. Create a loader function with a promise in it:
function LoadCSS( cssURL ) {
// 'cssURL' is the stylesheet's URL, i.e. /css/styles.css
return new Promise( function( resolve, reject ) {
var link = document.createElement( 'link' );
link.rel = 'stylesheet';
link.href = cssURL;
document.head.appendChild( link );
link.onload = function() {
resolve();
console.log( 'CSS has loaded!' );
};
} );
}
Then obviously you want something done after the CSS has loaded. You can call the function that needs to run after CSS has loaded like this:
LoadCSS( 'css/styles.css' ).then( function() {
console.log( 'Another function is triggered after CSS had been loaded.' );
return DoAfterCSSHasLoaded();
} );
Useful links if you want to understand in-depth how it works:
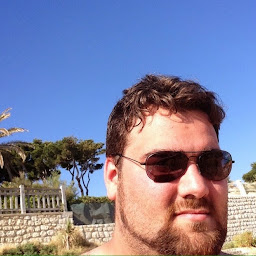
Comments
-
gregory boero.teyssier almost 2 years
Is it possible to import css stylesheets into a html page using Javascript? If so, how can it be done?
P.S the javascript will be hosted on my site, but I want users to be able to put in the
<head>
tag of their website, and it should be able to import a css file hosted on my server into the current web page. (both the css file and the javascript file will be hosted on my server).