How to make a child thread sleep without freezing UI thread in WPF application?
Solution 1
Have you tried it? Sleeping thread inside a Task won't freeze your GUI
but it will make the current background thread to go in sleep mode and your UI will remain responsive always -
Task.Factory.StartNew(() =>
{
Thread.Sleep(5000000); // this line won't make UI freeze.
});
Thread.Sleep(5000000); // But this will certainly do.
Solution 2
Since you're already using async
, you can just do it the asynchronous way:
var words = await Task.Run(async () =>
{
StringMgr.TextToUniqueWords(File.ReadAllText(filename));
await Task.Delay(TimeSpan.FromSeconds(10));
});
However, I suspect that sleeping is the wrong solution for the actual problem you're trying to solve. If you'd like to post another question with your actual problem, you may find a better solution.
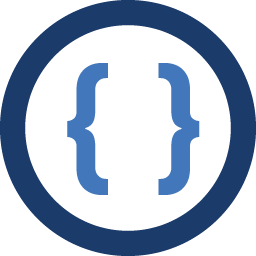
Admin
Updated on June 17, 2022Comments
-
Admin almost 2 years
I have a WPF application and I have individual threads running to accomplish tasks and I would like to have the current thread to go to sleep something like,
Thread.CurrentThread.Sleep(10000)
I do see it in Java but not in C#. I do know that I only have only one UI thread, so if I use Thread.Sleep(10000) my UI thread will be block. I am using async and await from .NET 4.5.
var words = await Task.Factory.StartNew(() => { StringMgr.TextToUniqueWords(File.ReadAllText(filename));
// I want to be able to sleep this thread for 10 seconds, without the UI freezing
});
So how do I put a child thread to sleep without freezing the UI thread in a WPF application?
Thanks!