How to make a JSON String using the key/value from the Map
Solution 1
Not sure what exact task you're working, but there are many JSON libraries for Java which can do this for you such as json in java and google-gson. For example, in json in java, once you have filled all the values in its JSONObject, then converting it into JSON string is fairly easy:
JSONObject.toString(); // compact JSON string
JSONObject.toString(int indent); // easier readable format with indention.
For example, in your case, you may create a JSONObject like the following and invoke its toString() function. This can safe your time from formatting string to fit the JSON format:
JSONObject jsonObject = new JSONObject();
Map<String, String> strStrMap = new HashMap<String, String>();
strStrMap.put("hello", "world");
strStrMap.put("here is", "an example");
jsonObject.put("myMap", strStrMap);
System.out.println(jsonObject.toString(2));
and here is its output:
{"myMap": {
"hello": "world",
"here is": "an example"
}}
Solution 2
You can just do something like this:
import org.json.JSONObject;
JSONObject rootJo = new JSONObject();
for (AttributeValue<?> al : list) {
JSONObject mapJo = new JSONObject(al.getValue());
rootJo.put("v", mapJo);
}
In other words:
- create a new (empty) JSONObject named rootJo (root JSON object)
- iterate over your list of maps
- for each element in the list, convert the map into a JSONObject (using a library)
- add a new property to rootJo (the key will be
v
) - set the value of
v
to the JSONObject that was created from the map
Maybe I'm missing something, but I would replace your entire method with this:
/**
* Creates a JSON object from a list of attributes that
* have maps as their values.
*/
private JSONObject toJson(List<AttributeValue<?>> attList) {
if (attList == null) {
return null;
}
JSONObject jo = new JSONObject();
JSONArray ja = new JSONArray();
for (AttributeValue<?> att : attList) {
JSONObject mapJo = new JSONObject(att.getValue());
ja.put("v", mapJo);
}
jo.put("lv", ja);
return jo;
}
You should also make it typesafe, but I'll leave the finer details to you...
(plus I'm not sure what an AttributeValue is... can't find it anywhere)
Solution 3
Use Jackson. It's lightweight and faster than Gson. It also isn't known to be bundled by OEMs, which can mess with your classpath.
Solution 4
When using google gson.
var getRowData =
[{
"dayOfWeek": "Sun",
"date": "11-Mar-2012",
"los": "1",
"specialEvent": "",
"lrv": "0"
},
{
"dayOfWeek": "Mon",
"date": "",
"los": "2",
"specialEvent": "",
"lrv": "0.16"
}];
JsonElement root = new JsonParser().parse(request.getParameter("getRowData"));
JsonArray jsonArray = root.getAsJsonArray();
JsonObject jsonObject1 = jsonArray.get(0).getAsJsonObject();
String dayOfWeek = jsonObject1.get("dayOfWeek").toString();
// when using jackson
JsonFactory f = new JsonFactory();
ObjectMapper mapper = new ObjectMapper();
JsonParser jp = f.createJsonParser(getRowData);
// advance stream to START_ARRAY first:
jp.nextToken();
// and then each time, advance to opening START_OBJECT
while (jp.nextToken() == JsonToken.START_OBJECT) {
Map<String,Object> userData = mapper.readValue(jp, Map.class);
userData.get("dayOfWeek");
// process
// after binding, stream points to closing END_OBJECT
}
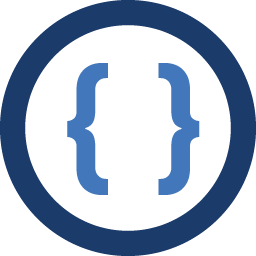
Admin
Updated on April 22, 2020Comments
-
Admin about 4 years
I am trying to create a JSON String using the corresponding key/value pair. In the below code, I am trying to iterate the list of
AttributeValue
and then I am trying to makie the JSON String using theal.getValue map
.private <T> String createJsonWithEscCharacters(List<AttributeValue<T>> list) { StringBuilder keyValue = new StringBuilder(); if (list != null) { for (AttributeValue<?> al: list) { keyValue.append("\"").append("v").append("\"").append(":").append(" {"); for (Map.Entry<String, String> entry : ((Map<String, String>) al.getValue()).entrySet()) { keyValue.append("\"").append(entry.getKey()).append("\""); keyValue.append(":").append(" \"").append(entry.getValue()).append("\"").append(","); System.out.println(keyValue); } } } return null; }
When I inspect on
al
, I seevalue
asLinkedHashMap<K,V>
and when I printal.getValue()
, it gives me this-{predictedCatRev=0;101;1,1;201;2, predictedOvrallRev=77;2,0;1,16;3, sitePrftblty=77;2,0;1671679, topByrGms=12345.67, usrCurncy=1, vbsTopByrGmb=167167.67}
So that means, I can iterate the
al.getValue()
map and use thosekey/value pair
to make the JSON String.Now I am trying to make a JSON String by iterating the
al.getValue()
map. So the JSON String should look something like this after iterating theal.getValue()
map-{ "lv": [ { "v": { "predictedCatRev": "0;101;1,1;201;2", "predictedOvrallRev": "77;2,0;1,16;3", "sitePrftblty": "77;2,0;1671679", "topByrGms": "12345.67", "usrCurncy": "1", "vbsTopByrGmb": "167167.67" } } ], }
I am wondering what is the cleanest way to do this? In my above code, I am not fully able to make the above JSON String but whatever code I have above, it is able to make slight portion of JSON String in the way I needed but the not full JSON String in the way, I am looking for. Can anyone help me on this like what will be the cleanest way to do this?
Thanks