How to make Horizontal scrollView and some buttons in it (programming)
Solution 1
I have tried your code and changed it a bit. It works as expected, unless you have something other going on:
var scView:UIScrollView!
let buttonPadding:CGFloat = 10
var xOffset:CGFloat = 10
override func viewDidLoad() {
super.viewDidLoad()
scView = UIScrollView(frame: CGRect(x: 0, y: 120, width: view.bounds.width, height: 50))
view.addSubview(scView)
scView.backgroundColor = UIColor.blue
scView.translatesAutoresizingMaskIntoConstraints = false
for i in 0 ... 10 {
let button = UIButton()
button.tag = i
button.backgroundColor = UIColor.darkGray
button.setTitle("\(i)", for: .normal)
//button.addTarget(self, action: #selector(btnTouch), for: UIControlEvents.touchUpInside)
button.frame = CGRect(x: xOffset, y: CGFloat(buttonPadding), width: 70, height: 30)
xOffset = xOffset + CGFloat(buttonPadding) + button.frame.size.width
scView.addSubview(button)
}
scView.contentSize = CGSize(width: xOffset, height: scView.frame.height)
}
Solution 2
There are two ways of achieving it :) One the hard way using scrollView and calculating offset and setting views programmatically on ScrollView as subView else use CollectionView
Step 1:
Add a UICollectionView to storyboard, set the height of collectionView to match your requirement :)
Step 2
Create a cell, size of which depends on your requirement. I have created a cell with background colour orange/pink. Added a label on it to show your number.
Step 3:
Set the reusable cell identifier to the cell and set its class as well :)
Step 4 :
Set collectionView scroll direction to horizontal :)
Step 5:
Now implement collectionView delegates and data source methods :)
extension ViewController : UICollectionViewDataSource,UICollectionViewDelegate {
func numberOfSections(in collectionView: UICollectionView) -> Int {
return 1
}
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 10
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "myCell", for: indexPath) as! MyCollectionViewCell
cell.myLabel.text = "ABCD"
return cell;
}
}
Thats all :)
Final O/P
Look at collectionView with cell ABCD :D
Additional Info
If you are dragging a collectionView on UIViewController, you might see that the collectionView leaves a gap at the top which you can solve by unchecking Adjust ScrollView Insets of ViewController :)
Solution 3
import UIKit
class ViewController: UIViewController {
var imageStrings:[String] = []
var myScrollView:UIScrollView!
@IBOutlet weak var previewView: UIImageView!
var scrollWidth : CGFloat = 320
let scrollHeight : CGFloat = 100
let thumbNailWidth : CGFloat = 80
let thumbNailHeight : CGFloat = 80
let padding: CGFloat = 10
override func viewDidLoad() {
super.viewDidLoad()
imageStrings = ["image01","image02","image03","image04","image05","image06","image07","image08"]
scrollWidth = self.view.frame.width
//setup scrollView
myScrollView = UIScrollView(frame: CGRectMake(0, self.view.frame.height - scrollHeight, scrollWidth, scrollHeight))
//setup content size for scroll view
let contentSizeWidth:CGFloat = (thumbNailWidth + padding) * (CGFloat(imageStrings.count))
let contentSize = CGSize(width: contentSizeWidth ,height: thumbNailHeight)
myScrollView.contentSize = contentSize
myScrollView.autoresizingMask = UIViewAutoresizing.FlexibleWidth
for(index,value) in enumerate(imageStrings) {
var button:UIButton = UIButton.buttonWithType(.Custom) as! UIButton
//calculate x for uibutton
var xButton = CGFloat(padding * (CGFloat(index) + 1) + (CGFloat(index) * thumbNailWidth))
button.frame = CGRectMake(xButton,padding, thumbNailWidth, thumbNailHeight)
button.tag = index
let image = UIImage(named:value)
button.setBackgroundImage(image, forState: .Normal)
button.addTarget(self, action: Selector("changeImage:"), forControlEvents: .TouchUpInside)
myScrollView.addSubview(button)
}
previewView.image = UIImage(named: imageStrings[0])
self.view.addSubview(myScrollView)
}
// Do any additional setup after loading the view, typically from a nib.
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func changeImage(sender:UIButton){
let name = imageStrings[sender.tag]
previewView.image = UIImage(named: name)
}
}
Solution 4
Its easy with minimal use of storyboard. You can avoid that rather easily. All you need are:
- Scroll view
- Horizontal stack view
- Set the constraints of the above as shown in the project
- A number of buttons - as many view controllers that need to be displayed.
- A container view - this will be the view that will hold the active view controller.
Please refer to this project for some sample code: https://github.com/equitronix/horizontalScrollMenu
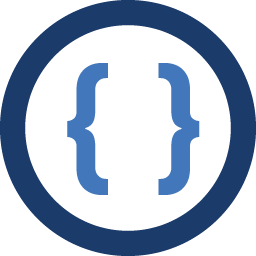
Admin
Updated on October 18, 2020Comments
-
Admin over 3 years
sorry,I have a problem.I don't use storyBoard.
I want to make a view like this.
photo by terenceLuffy/AppStoreStyleHorizontalScrollViewbut I try to do this. Finlly,scrollView just show last button like this.
This is code:
var scView:UIScrollView = UIScrollView() var buttonPadding:CGFloat = 10 var xOffset:CGFloat = 10 scView.backgroundColor = UIColor.blue scView.translatesAutoresizingMaskIntoConstraints = false func viewDidLoad() { for i in 0 ... 10 { let button = UIButton() button.tag = i button.backgroundColor = UIColor.darkGray button.setTitle("\(i)", for: .normal) button.addTarget(self, action: #selector(btnTouch), for: UIControlEvents.touchUpInside) button.frame = CGRect(x: xOffset, y: CGFloat(buttonPadding), width: 70, height: 30) xOffset = xOffset + CGFloat(buttonPadding) + button.frame.size.width scView.addSubview(button) } scView.contentSize = CGSize(width: xOffset, height: scView.frame.height) }
please help me. Thanks all!
-
Admin over 7 yearsthanks,but i just want to use scrollView to show my view.
-
Rick over 6 yearsWelcome to Stack Overflow. Please review How do I write a good answer.