How to make HTTP call from Controller ? to Use web API's Asp.Net Core C#
25,596
Solution 1
Use ajax to call controler:
$.ajax({
type: "GET",
url: "/API/Gumtreetoken?user=username&pasword=password",
success: function (atsakas) {
alert(atsakas);
},
error: function (error) {
alert("error");
}
});
And, from controller I use HTTPClient to make POST call and get needed values from XML responce.
[Authorize]
[Route("API/Gumtreetoken")]
public IActionResult GumtreePost(string user, string pasword)
{
string atsakas = "";
string token = "";
string id = "";
using (HttpClient client = new HttpClient())
{
//parametrai (PARAMS of your call)
var parameters = new Dictionary<string, string> { { "username", "YOURUSERNAME" }, { "password", "YOURPASSWORD" } };
//Uzkoduojama URL'ui
var encodedContent = new FormUrlEncodedContent(parameters);
try
{
//Post http callas.
HttpResponseMessage response = client.PostAsync("https://feed-api.gumtree.com/api/users/login", encodedContent).Result;
//nesekmes atveju error..
response.EnsureSuccessStatusCode();
//responsas to string
string responseBody = response.Content.ReadAsStringAsync().Result;
atsakas = responseBody;
}
catch (HttpRequestException e)
{
Console.WriteLine("\nException Caught!");
Console.WriteLine("Message :{0} ", e.Message);
}
//xml perskaitymui
XmlDocument doc = new XmlDocument();
//xml uzpildomas api atsakymu
doc.LoadXml(@atsakas);
//iesko TOKEN
XmlNodeList xmltoken = doc.GetElementsByTagName("user:token");
//iesko ID
XmlNodeList xmlid = doc.GetElementsByTagName("user:id");
token = xmltoken[0].InnerText;
id = xmlid[0].InnerText;
atsakas = "ID: " + id + " Token: " + token;
}
return Json(atsakas);
}
This should be Async, so, you could do like this:
[Authorize]
[Route("API/Gumtreetoken")]
public async Task<IActionResult> GumtreePost(string user, string pasword)
{
string atsakas = "";
string token = "";
string id = "";
using (HttpClient client = new HttpClient())
{
var parameters = new Dictionary<string, string> { { "username", "YOURUSERNAME" }, { "password", "YOURPASSWORD" } };
var encodedContent = new FormUrlEncodedContent(parameters);
try
{
HttpResponseMessage response = await client.PostAsync("https://feed-api.gumtree.com/api/users/login", encodedContent);
response.EnsureSuccessStatusCode();
string responseBody = await response.Content.ReadAsStringAsync();
atsakas = responseBody;
}
catch (HttpRequestException e)
{
Console.WriteLine("\nException Caught!");
Console.WriteLine("Message :{0} ", e.Message);
}
XmlDocument doc = new XmlDocument();
doc.LoadXml(@atsakas);
XmlNodeList xmltoken = doc.GetElementsByTagName("user:token");
XmlNodeList xmlid = doc.GetElementsByTagName("user:id");
token = xmltoken[0].InnerText;
id = xmlid[0].InnerText;
atsakas = "ID: " + id + " Token: " + token;
}
return Json(atsakas);
}
Solution 2
Try this code: To make Api call from Asp.Net Core, Server Side (C#).
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net.Http;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
namespace core.api.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ValuesController : ControllerBase
{
[HttpGet]
public async Task<ActionResult<string>> Get()
{
string url="https://jsonplaceholder.typicode.com/todos"; // sample url
using (HttpClient client = new HttpClient())
{
return await client.GetStringAsync(url);
}
}
}
}
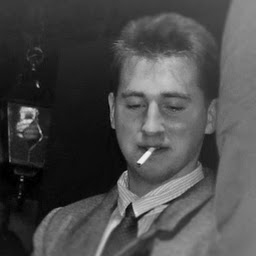
Author by
Emilis Vadopalas
.NET Developer Familiar with: C# (MVC, Blazor/Razor, API's, Forms, WPF, Core, Framework 4) Databases (MySQL,MSSQL) JavaScript (ES5, ES6+, Typescript, React) HTML/CSS Conteiners (Docker)
Updated on April 17, 2021Comments
-
Emilis Vadopalas about 3 years
I want to make HTTP calls to various services, POST/GET/DELETE.., and read responses JSON AND XML, How do I do this in C# on servers side?
In short: How to make Api call from Asp.Net Core C#.
Client side Ajax doesn't work, (for cross domains)
-
Nithin Paul over 2 yearsCan you help me here stackoverflow.com/questions/69806158/…