How to make service run even in sleep mode?
If you want to ensure that your service is not killed/reclaimed by the OS you need to make it a foreground service. By default all services are background, meaning they will be killed when the OS needs resources. For details refer to this doc
Basically, you need to create a Notification
for your service and indicate that it is foreground. This way the user will see a persistent notification so he knows your app is running, and the OS will not kill your service.
Here is a simple example of how to create a notification (do this in your service) and make it foreground:
Intent intent = new Intent(this, typeof(SomeActivityInYourApp));
PendingIntent pi = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT);
NotificationCompat.Builder builder = new NotificationCompat.Builder(this);
builder.setSmallIcon(Resource.Drawable.my_icon);
builder.setTicker("App info string");
builder.setContentIntent(pi);
builder.setOngoing(true);
builder.setOnlyAlertOnce(true);
Notification notification = builder.build();
// optionally set a custom view
startForeground(SERVICE_NOTIFICATION_ID, notification);
Please note that the above example is rudimentary and does not contain code to cancel the notification, amongst other things. Also, when your app no longer needs the service it should call stopForeground
in order to remove the notification and allow your service to be killed, not doing so is a waste of resources.
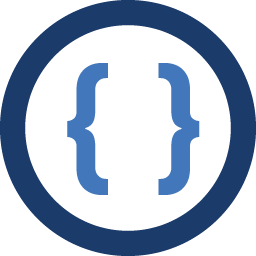
Admin
Updated on June 21, 2022Comments
-
Admin almost 2 years
I have service that is implementing location listener. Now my question is how to make sure that my service will capture location even in sleep mode. I have read about alarm manager
alarm.setRepeating(AlarmManager.RTC_WAKEUP, triggerAtMillis, intervalMillis, operation);
but how to use it. here is my code.. any help would be appreciated..
my servicepublic class LocationCaptureService extends Service implements LocationListener { public static int inteval; java.sql.Timestamp createdTime; LocationManager LocationMngr; @Override public void onCreate() { inteval=10*1000; startLocationListener(inteval); } @Override public IBinder onBind(Intent arg0) { return null; } private void startLocationListener(int inteval,String nwProvider) { this.LocationMngr = (LocationManager)getSystemService(Context.LOCATION_SERVICE); this.LocationMngr.requestLocationUpdates(LocationManager.GPS_PROVIDER, inteval, 0, this); } public void onLocationChanged(Location location) { String status="c",S=null; double longitude,lattitude,altitude; g_currentBestLocation = location; createdTime = new Timestamp (new java.util.Date().getTime()); longitude=location.getLongitude(); lattitude=location.getLatitude(); altitude=location.getAltitude(); //use this } } public void onProviderDisabled(String provider) {} public void onProviderEnabled(String provider) {} public void onStatusChanged(String provider, int status, Bundle extras) {}
}