How to make SwiftUI view fullscreen?
Solution 1
Just swap the ..background(Color.red) and .edgesIgnoringSafeArea(.all). And it will work perfectly.
struct ContentView : View {
var body: some View {
Text("Test")
.frame(minWidth: 0, maxWidth: .infinity, minHeight: 0, maxHeight: .infinity)
.background(Color.red)
.edgesIgnoringSafeArea(.all)
}
}
Solution 2
The Augmented Reality App with SwiftUI also has a problem in entering full screen. (Xcode 12.3, SwiftUI 5.3)
struct ContentView : View {
var body: some View {
return ARViewContainer().edgesIgnoringSafeArea(.all)
}
}
The code above is from the AR template. However, it doesn't work.
The solution is tricky: you have to set "LaunchScreen.storyboard" as "Launch Screen File" in "[yourTarget] -> General -> App Icons and Launch Images". If there is no "LaunchScreen.storyboard" in the project, it can still work by entering "LaunchScreen" in that field.
I found a similar discussion on Apple forum that explains the potential reason of failure:
If that isn't set properly, then it sounds like your app is launching into a compatibility mode which is letterboxing your app.
Solution 3
According to Apple documentation from iOS 14 you can use fullScreenCover(item:onDismiss:content:)
And here is sample code:
struct ContentView: View {
@State private var isFullScreen = false
var body: some View {
ZStack{
Color.yellow.edgesIgnoringSafeArea(.all)
Text("Hello, FullScreen!")
.padding()
.background(Color.blue)
.foregroundColor(.green)
.cornerRadius(8)
.fullScreenCover(isPresented: $isFullScreen) {
FullScreen(isFullScreen: $isFullScreen)
}
.onTapGesture {
isFullScreen.toggle()
}
}
}
}
struct FullScreen: View {
@Binding var isFullScreen: Bool
var body: some View {
ZStack {
Color.red.edgesIgnoringSafeArea(.all)
Text("This is full screen!!")
.onTapGesture {
self.isFullScreen.toggle()
}
}
}
}
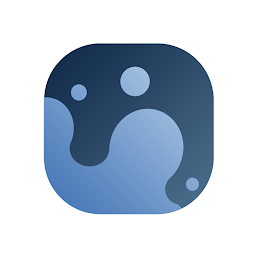
timfraedrich
Updated on December 23, 2021Comments
-
timfraedrich over 2 years
As the title already says I'm trying to make a view fullscreen (make it extend over the
SafeArea
), butSwiftUI
seems to always align views to thesafeArea
.After researching this for a while I found
.edgesIgnoringSafeArea(.all)
which seems like a pretty straightforward way to do it. The problem is that it doesn't work. The view still isn't full screen. Here is some example code:struct ContentView : View { var body: some View { Text("Test") .frame(minWidth: 0, maxWidth: .infinity, minHeight: 0, maxHeight: .infinity) .edgesIgnoringSafeArea(.all) .background(Color.red) } }
-
timfraedrich almost 5 yearsthat's weird, I actually tried that already, but now it seems to work. Maybe it's just a glitch in the beta. Thanks anyway.
-
Sulthan almost 5 yearsIs that a bug or is that how it's supposed to work?
-
Md Shafiul Islam almost 5 yearsMay be it's a bug or may be not. I can't say for sure. But I realized that if I give a background color after Text("Test"), it will only color the content frame the string "Test" takes. But if I give a background color after setting a frame then it will color the whole frame.
-
mbxDev almost 5 yearsI think it's a bug...
.edgesIgnoringSafeArea(.all)
should modify the size of the View, and.background(Color.red)
should modify the background color of the View. Instead, it seems that.background()
is also modifying the size. -
CMash over 4 yearsIt's not a bug, it gives you flexibility over what you want to happen, though is quite confusing an awkward in a way. Basically the colour applies to the view before it's resized by any subsequent modifiers. Apply the colour first and then something like padding and the extra padding isn't coloured. Do the padding first and then colour and you get the full size view coloured.
-
claude31 over 4 yearsIt behaves as if there were several layers of views, creating a new view after padding. Is it the case ? Consider the following: var body: some View { Text("Test") .frame(minWidth: 0, maxWidth: .infinity, minHeight: 0, maxHeight: .infinity) .edgesIgnoringSafeArea(.all) .background(Color.red) .padding(25) .background(Color.blue) }. that give a red content with a blue border and not a full blue.
-
LukeSideWalker over 3 yearsWell not really a solution for sheets in full-size, though a very nice solution for the underlying use case. Thanks a lot, appreciate your piece of code.
-
James Castrejon about 3 yearsI had the same issue but I was using SwiftUI and SpriteKit. For whatever reason, it would not go into fullscreen. I tried this even though I don't have a launch screen file and it worked. Spent hours scratching my head over this. I'm glad it's working now though.