How to mock application context
13,646
As a base I would use the Mockito annotations( i assume you want to mock the view also):
public class TutorProfilePresenter{
@InjectMocks
private TutorProfilePresenter presenter;
@Mock
private TutorProfileScreenView viewMock;
@Mock
private Context contextMock;
@Before
public void init(){
MockitoAnnotations.initMocks(this);
}
@Test
public void test() throws Exception{
// configure mocks
when(contextMock.someMethod()).thenReturn(someValue);
// call method on presenter
// verify
verify(viewMock).setBasePrice(someNumber...)
}
}
This would inject ready to configure mocks into your class under test.
More insight on Mockito stubbing: sourceartists.com/mockito-stubbing
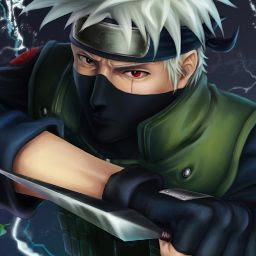
Author by
Jay kishan
Updated on June 24, 2022Comments
-
Jay kishan almost 2 years
How do we mock an Application Context? I got a presenter which I intend to write a test for. The parameters it receives were a
view
andContext
. How do I create a mock for context to work?public TutorProfilePresenter(TutorProfileScreenView view, Context context){ this.view = view; this.context = context } public void setPrice(float price,int selectedTopics){ int topicsPrice = 0; if(selectedTopics>2) { topicsPrice = (int) ((price/5.0)*(selectedTopics-2)); } view.setBasePrice(price,topicsPrice,selectedTopics, price+topicsPrice); }