How to open .pbtxt file?
12,634
Solution 1
i.e your pbtxt file name is graphfilename.pbtxt
Example
import tensorflow as tf
from tensorflow.core.framework import graph_pb2 as gpb
from google.protobuf import text_format as pbtf
gdef = gpb.GraphDef()
with open('graphfilename.pbtxt', 'r') as fh:
graph_str = fh.read()
pbtf.Parse(graph_str, gdef)
tf.import_graph_def(gdef)
Solution 2
Here is a solution to read the label_map.pbtxt file. It doesn't require any protobuf imports, so it works across all versions of TF.
def read_label_map(label_map_path):
item_id = None
item_name = None
items = {}
with open(label_map_path, "r") as file:
for line in file:
line.replace(" ", "")
if line == "item{":
pass
elif line == "}":
pass
elif "id" in line:
item_id = int(line.split(":", 1)[1].strip())
elif "name" in line:
item_name = line.split(":", 1)[1].replace("'", "").strip()
if item_id is not None and item_name is not None:
items[item_name] = item_id
item_id = None
item_name = None
return items
print ([i for i in read_label_map("label_map.pbtxt")])
Solution 3
Just modified the @leenremm's code as it wasnt working out for me!
def read_label_map(label_map_path):
item_id = None
item_name = None
items = {}
with open(label_map_path, "r") as file:
for line in file:
line.replace(" ", "")
if line == "item{":
pass
elif line == "}":
pass
elif "id" in line:
item_id = int(line.split(":", 1)[1].strip())
elif "display_name" in line:
item_name = line.split(" ")[-1].replace("\"", " ").strip()
if item_id is not None and item_name is not None:
items[item_id] = item_name
item_id = None
item_name = None
return items
print(read_label_map(pbtxt_path))
The function will return a dictionary where you can easily "get()" the category names by the category id.
label_map = read_label_map(label_map_path)
label_map.get(2)
Solution 4
You can open it in any text editor, like Sublime Text or whatever your OS has to offer by default.
If you are on a Linux/macOS system, you can also open it in Terminal by directing to its directory and writing
nano {filename}.pbtxt
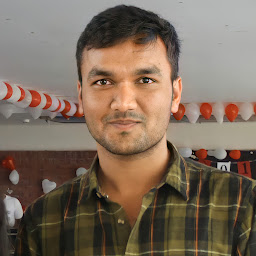
Comments
-
Harshal Deore almost 2 years
Trying to change the labels from the tensorflow object detection model but Not able to open pbtxt file. Can tell me if there is any application to open it?
-
Onkar Musale about 5 yearsPossible duplicate of open tensorflow graph from file
-
GPhilo about 5 years@OnkarMusale the labels pbtxt file in the object detection API is not a TF Graph, but a label map (see MSCOCO example here). The OP doesn't need to load the graph, but literally just open the text file and modify it
-
-
YoungChul about 5 yearsI think you're misunderstanding the question. He is simply asking about how to open the label file for training a Tensorflow model. Which can be done with a simple text editor.
-
Achyut Sarma about 3 yearsThis is exactly what I was looking for. Thanks, man for making it so simple.
-
BaseballR over 2 yearscame here and used this. One note, if your IDs include id or name in them this won't work. I added id: and name: and worked for me, but just make sure your data doesn't have that in it.